In an set of integers, find three elements summing to zero (3-sum, leetcode variant) The Next...
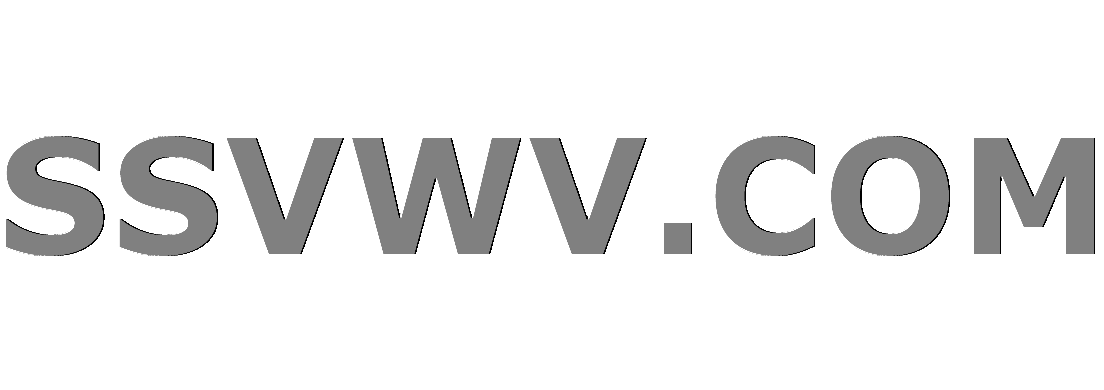
Multi tool use
How to start emacs in "nothing" mode (`fundamental-mode`)
"and that skill is always a class skill for you" - does "always" have any meaning in Pathfinder?
Workaholic Formal/Informal
What flight has the highest ratio of time difference to flight time?
How do I go from 300 unfinished/half written blog posts, to published posts?
What exact does MIB represent in SNMP? How is it different from OID?
Giving the same color to different shapefiles in QGIS
Opposite of a diet
Does it take more energy to get to Venus or to Mars?
Hindi speaking tourist to UK from India
How to make a variable always equal to the result of some calculations?
Is there a way to save my career from absolute disaster?
In excess I'm lethal
Different harmonic changes implied by a simple descending scale
If Nick Fury and Coulson already knew about aliens (Kree and Skrull) why did they wait until Thor's appearance to start making weapons?
Is it ever safe to open a suspicious html file (e.g. email attachment)?
What is the purpose of the Evocation wizard's Potent Cantrip feature?
Science fiction short story involving a paper written by a schizophrenic
Disadvantage of gaining multiple levels at once in a short milestone-XP game
How to log in to Centos 7 using RDP from Win10
If the heap is initialized for security, then why is the stack uninitialized?
Do I need to enable Dev Hub in my PROD Org?
How long to clear the 'suck zone' of a turbofan after start is initiated?
What does convergence in distribution "in the Gromov–Hausdorff" sense mean?
In an set of integers, find three elements summing to zero (3-sum, leetcode variant)
The Next CEO of Stack OverflowGiven an array find any three numbers which sum to zero3-Sum Problem in PythonGiven a vector and a target sum, returns zero-based indices of any two distinct elements whose sum is equal to the target sumPython 3 two-sum performanceFind the pairs of integers that sum to NFind contiguous integers with a given sumFind all combinations of 4 elements whose sum equals a target in PythonLeetcode Mountain ArrayLeetcode Three Sum in PythonHash table solution to twoSum
$begingroup$
I use a dummy solution to solve the leetcode 3Sum problem.
I employ the set data type to handle duplicates, then transform back to list.
Given an array
nums
of n integers, are there elements a, b, c innums
such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.
Note:
The solution set must not contain duplicate triplets.
Example:
Given array
nums = [-1, 0, 1, 2, -1, -4]
,
A solution set is:
[
[-1, 0, 1],
[-1, -1, 2]
]
My codes:
from typing import List
import logging
import unittest
import random
from collections import defaultdict,Counter
##logging.disable(level=#logging.CRITICAL)
##logging.basicConfig(level=#logging.DEBUG, format="%(levelname)s %(message)s")
class Solution:
def threeSum(self, nums, target: int=0) -> List[List[int]]:
"""
:type nums: List[int]
:type target: int
"""
if len(nums) < 3: return []
triplets = []
if target == [0, 0, 0]:
triplets.append([0, 0, 0])
return triplets # finish fast
lookup = {nums[i]:i for i in range(len(nums))} #overwrite from the high
if len(lookup) == 1:#assert one identical element
keys = [k for k in lookup.keys()]
if keys[0] != 0:
return []
else:
triplets.append([0,0,0])
return triplets
triplets_set = set()
for i in range(len(nums)):
num_1 = nums[i]
sub_target = target - num_1
# #logging.debug(f"level_1_lookup: {lookup}")
for j in range(i+1, len(nums)):
num_2 = nums[j]
num_3 = sub_target - num_2
k = lookup.get(num_3) #
if k not in {None, i, j}: #don't reproduce itself
result = [num_1, num_2, num_3]
result.sort()
result = tuple(result)
triplets_set.add(result)
triplets = [list(t) for t in triplets_set]
return triplets
My solution gets this result:
Your runtime beats 28.86 % of python3 submissions.
Could anyone please give hints to improve?
python performance algorithm programming-challenge k-sum
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I use a dummy solution to solve the leetcode 3Sum problem.
I employ the set data type to handle duplicates, then transform back to list.
Given an array
nums
of n integers, are there elements a, b, c innums
such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.
Note:
The solution set must not contain duplicate triplets.
Example:
Given array
nums = [-1, 0, 1, 2, -1, -4]
,
A solution set is:
[
[-1, 0, 1],
[-1, -1, 2]
]
My codes:
from typing import List
import logging
import unittest
import random
from collections import defaultdict,Counter
##logging.disable(level=#logging.CRITICAL)
##logging.basicConfig(level=#logging.DEBUG, format="%(levelname)s %(message)s")
class Solution:
def threeSum(self, nums, target: int=0) -> List[List[int]]:
"""
:type nums: List[int]
:type target: int
"""
if len(nums) < 3: return []
triplets = []
if target == [0, 0, 0]:
triplets.append([0, 0, 0])
return triplets # finish fast
lookup = {nums[i]:i for i in range(len(nums))} #overwrite from the high
if len(lookup) == 1:#assert one identical element
keys = [k for k in lookup.keys()]
if keys[0] != 0:
return []
else:
triplets.append([0,0,0])
return triplets
triplets_set = set()
for i in range(len(nums)):
num_1 = nums[i]
sub_target = target - num_1
# #logging.debug(f"level_1_lookup: {lookup}")
for j in range(i+1, len(nums)):
num_2 = nums[j]
num_3 = sub_target - num_2
k = lookup.get(num_3) #
if k not in {None, i, j}: #don't reproduce itself
result = [num_1, num_2, num_3]
result.sort()
result = tuple(result)
triplets_set.add(result)
triplets = [list(t) for t in triplets_set]
return triplets
My solution gets this result:
Your runtime beats 28.86 % of python3 submissions.
Could anyone please give hints to improve?
python performance algorithm programming-challenge k-sum
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
1
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
1
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How couldtarget: int
== [0, 0, 0]
?
$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
Ah, before I forget again: Congrats on asking for a review, forhints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!
$endgroup$
– greybeard
yesterday
add a comment |
$begingroup$
I use a dummy solution to solve the leetcode 3Sum problem.
I employ the set data type to handle duplicates, then transform back to list.
Given an array
nums
of n integers, are there elements a, b, c innums
such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.
Note:
The solution set must not contain duplicate triplets.
Example:
Given array
nums = [-1, 0, 1, 2, -1, -4]
,
A solution set is:
[
[-1, 0, 1],
[-1, -1, 2]
]
My codes:
from typing import List
import logging
import unittest
import random
from collections import defaultdict,Counter
##logging.disable(level=#logging.CRITICAL)
##logging.basicConfig(level=#logging.DEBUG, format="%(levelname)s %(message)s")
class Solution:
def threeSum(self, nums, target: int=0) -> List[List[int]]:
"""
:type nums: List[int]
:type target: int
"""
if len(nums) < 3: return []
triplets = []
if target == [0, 0, 0]:
triplets.append([0, 0, 0])
return triplets # finish fast
lookup = {nums[i]:i for i in range(len(nums))} #overwrite from the high
if len(lookup) == 1:#assert one identical element
keys = [k for k in lookup.keys()]
if keys[0] != 0:
return []
else:
triplets.append([0,0,0])
return triplets
triplets_set = set()
for i in range(len(nums)):
num_1 = nums[i]
sub_target = target - num_1
# #logging.debug(f"level_1_lookup: {lookup}")
for j in range(i+1, len(nums)):
num_2 = nums[j]
num_3 = sub_target - num_2
k = lookup.get(num_3) #
if k not in {None, i, j}: #don't reproduce itself
result = [num_1, num_2, num_3]
result.sort()
result = tuple(result)
triplets_set.add(result)
triplets = [list(t) for t in triplets_set]
return triplets
My solution gets this result:
Your runtime beats 28.86 % of python3 submissions.
Could anyone please give hints to improve?
python performance algorithm programming-challenge k-sum
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
I use a dummy solution to solve the leetcode 3Sum problem.
I employ the set data type to handle duplicates, then transform back to list.
Given an array
nums
of n integers, are there elements a, b, c innums
such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.
Note:
The solution set must not contain duplicate triplets.
Example:
Given array
nums = [-1, 0, 1, 2, -1, -4]
,
A solution set is:
[
[-1, 0, 1],
[-1, -1, 2]
]
My codes:
from typing import List
import logging
import unittest
import random
from collections import defaultdict,Counter
##logging.disable(level=#logging.CRITICAL)
##logging.basicConfig(level=#logging.DEBUG, format="%(levelname)s %(message)s")
class Solution:
def threeSum(self, nums, target: int=0) -> List[List[int]]:
"""
:type nums: List[int]
:type target: int
"""
if len(nums) < 3: return []
triplets = []
if target == [0, 0, 0]:
triplets.append([0, 0, 0])
return triplets # finish fast
lookup = {nums[i]:i for i in range(len(nums))} #overwrite from the high
if len(lookup) == 1:#assert one identical element
keys = [k for k in lookup.keys()]
if keys[0] != 0:
return []
else:
triplets.append([0,0,0])
return triplets
triplets_set = set()
for i in range(len(nums)):
num_1 = nums[i]
sub_target = target - num_1
# #logging.debug(f"level_1_lookup: {lookup}")
for j in range(i+1, len(nums)):
num_2 = nums[j]
num_3 = sub_target - num_2
k = lookup.get(num_3) #
if k not in {None, i, j}: #don't reproduce itself
result = [num_1, num_2, num_3]
result.sort()
result = tuple(result)
triplets_set.add(result)
triplets = [list(t) for t in triplets_set]
return triplets
My solution gets this result:
Your runtime beats 28.86 % of python3 submissions.
Could anyone please give hints to improve?
python performance algorithm programming-challenge k-sum
python performance algorithm programming-challenge k-sum
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited yesterday


Toby Speight
26.8k742118
26.8k742118
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Mar 26 at 3:28


AliceAlice
2164
2164
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Alice is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
1
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How couldtarget: int
== [0, 0, 0]
?
$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
Ah, before I forget again: Congrats on asking for a review, forhints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!
$endgroup$
– greybeard
yesterday
add a comment |
1
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
1
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How couldtarget: int
== [0, 0, 0]
?
$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
Ah, before I forget again: Congrats on asking for a review, forhints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!
$endgroup$
– greybeard
yesterday
1
1
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
1
1
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How could
target: int
== [0, 0, 0]
?$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How could
target: int
== [0, 0, 0]
?$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
Ah, before I forget again: Congrats on asking for a review, for
hints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!$endgroup$
– greybeard
yesterday
$begingroup$
Ah, before I forget again: Congrats on asking for a review, for
hints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!$endgroup$
– greybeard
yesterday
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
Multi-part answer: [0) these preliminaries] 1) review of code presented 2) hints for improvement excluding personal, from programming over python and accepting a programming challenge to k-sum and leetcode 3Sum.
One general principle to follow is do as expected, in programming, it has been formulated in many guises, including Principle of Least Surprise. Every other route leaves you under pressure to justify, at risk of being misunderstood.
In coding, document, in the program source code:
What is "everything" there for?
Such rules often have been gathered into guide lines, for Python, follow the Style Guide for Python Code.
- Instead of a docstring, your module starts with imports - most of them unused.
If your code mentioned leetcode, the presentation of aclass Solution
with just one function definition wouldn't beg justification.- the definition of
threeSum()
shows (a cute addition oftarget
&default and) a curious mix of leetcode's templates for Python 2&3, lacking a proper docstring - comparing "the int"
target
to the list literal[0, 0, 0]
is dangerous given Python's zeal to allow operations: do you know by heart when that evaluates toTrue
?
(Do you expect most every reader of your code to?)
(Belatedly, it occurred to me that you may have intended to comparenums
to[0, 0, 0]
- bogus iftarget != 0
) - verbosity -
you can denote a tuple containing a single literal list like([v, v, v],)
- 2nd early out:
with only one key→value in the dict, there's no need to access it:
every key will equalnums[0]
You need to check for3*nums[0] != target
- naming (Not your fault:
Solution
andthreeSum
are substandard.nums
/_1-3 don't feel bad enough to change):
-target
,triplets
,sub_target
: right on!
- given Python's "duck typing", I'd stick withtriplets
(, never introducingtriplets_set
)
-lookup
is a horrible name →value_to_index
(or, in a suitably small context, justindex
)
(- there's one thing wrong withk
: it gets in the way when extending three_sum() to k_sum()…)
pythonic or not (or exploiting library functions, rather?):value_to_index = { value: index for index, value in enumerate(nums) }
(same forfor i, num_1 in enumerate(nums)
,for j, num_2 in enumerate(nums[i+1:], i+1)
)- commenting
- you did comment, and I find half of the comments helpful
- I don't get#overwrite from the high
(that may just be me)
-#don't reproduce itself
: probablyuse any element/index once, at most
rather thandon't repeat an element/index
- you did not comment the outer for loop, arguably the most profitable place:
how is what the execution constructs a solution?:
for each value,value_to_index
keeps just the last index: how does this not prevent any valid triple to show up in the solution? - checking
k
: nice!
(I'd go forin (None, i, j)
)
you don't provide a "tinker harness"
if __name__ == '__main__':
object_disoriented = Solution()
print(object_disoriented.threeSum([-1, 0, 1, 2, -1, -4]))
print(object_disoriented.threeSum([1, 1, 1], 3))
(Something else to follow is make (judicious) use of every help you can: if you use an IDE supporting Python, chances are it can help you with PEP8.)
(Running low on steam, this part will start more frugal than expected.)
Real Programming is the creation of a language that simplifies the solution of the problem at hand.
With Python providing most mechanisms needed in programming challenges, that leaves:
- Code readably.
- Create elements easy to use correctly.
I bickered you some about having a consistent problem description.
This is another place where Test First shines:
As long as you find yourself not knowing what to test, you are not in a position to implement anything, yet. (Inconsistencies in specification tend to stand out in test design & implementation.)
Programming challenges à la leetcode.com typically build on simpler challenges - you met 2sum, which puts you in a favourable position to tackle 3sum.
The performance part of these challenges is about not doing things over, not discarding knowledge more often than about algebraic insight.
The generic solution to 2sum is to take values and find the complement (1sum?).
The extension to k-sum is to split k into smaller numbers.
Ways to have information about the order of a set or sequence (say, elements) of instances of a type with ordering reusable is to have them ordered (ordered = sorted(elements)
) or sorted (histogram = Counter(elements)
).
For 2sum, you could search for target/2
and work inside-out.
For 3sum, one value will be no greater, another no smaller than both others. The third one will not "lie outside" the former two.
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Alice is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216207%2fin-an-set-of-integers-find-three-elements-summing-to-zero-3-sum-leetcode-vari%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Multi-part answer: [0) these preliminaries] 1) review of code presented 2) hints for improvement excluding personal, from programming over python and accepting a programming challenge to k-sum and leetcode 3Sum.
One general principle to follow is do as expected, in programming, it has been formulated in many guises, including Principle of Least Surprise. Every other route leaves you under pressure to justify, at risk of being misunderstood.
In coding, document, in the program source code:
What is "everything" there for?
Such rules often have been gathered into guide lines, for Python, follow the Style Guide for Python Code.
- Instead of a docstring, your module starts with imports - most of them unused.
If your code mentioned leetcode, the presentation of aclass Solution
with just one function definition wouldn't beg justification.- the definition of
threeSum()
shows (a cute addition oftarget
&default and) a curious mix of leetcode's templates for Python 2&3, lacking a proper docstring - comparing "the int"
target
to the list literal[0, 0, 0]
is dangerous given Python's zeal to allow operations: do you know by heart when that evaluates toTrue
?
(Do you expect most every reader of your code to?)
(Belatedly, it occurred to me that you may have intended to comparenums
to[0, 0, 0]
- bogus iftarget != 0
) - verbosity -
you can denote a tuple containing a single literal list like([v, v, v],)
- 2nd early out:
with only one key→value in the dict, there's no need to access it:
every key will equalnums[0]
You need to check for3*nums[0] != target
- naming (Not your fault:
Solution
andthreeSum
are substandard.nums
/_1-3 don't feel bad enough to change):
-target
,triplets
,sub_target
: right on!
- given Python's "duck typing", I'd stick withtriplets
(, never introducingtriplets_set
)
-lookup
is a horrible name →value_to_index
(or, in a suitably small context, justindex
)
(- there's one thing wrong withk
: it gets in the way when extending three_sum() to k_sum()…)
pythonic or not (or exploiting library functions, rather?):value_to_index = { value: index for index, value in enumerate(nums) }
(same forfor i, num_1 in enumerate(nums)
,for j, num_2 in enumerate(nums[i+1:], i+1)
)- commenting
- you did comment, and I find half of the comments helpful
- I don't get#overwrite from the high
(that may just be me)
-#don't reproduce itself
: probablyuse any element/index once, at most
rather thandon't repeat an element/index
- you did not comment the outer for loop, arguably the most profitable place:
how is what the execution constructs a solution?:
for each value,value_to_index
keeps just the last index: how does this not prevent any valid triple to show up in the solution? - checking
k
: nice!
(I'd go forin (None, i, j)
)
you don't provide a "tinker harness"
if __name__ == '__main__':
object_disoriented = Solution()
print(object_disoriented.threeSum([-1, 0, 1, 2, -1, -4]))
print(object_disoriented.threeSum([1, 1, 1], 3))
(Something else to follow is make (judicious) use of every help you can: if you use an IDE supporting Python, chances are it can help you with PEP8.)
(Running low on steam, this part will start more frugal than expected.)
Real Programming is the creation of a language that simplifies the solution of the problem at hand.
With Python providing most mechanisms needed in programming challenges, that leaves:
- Code readably.
- Create elements easy to use correctly.
I bickered you some about having a consistent problem description.
This is another place where Test First shines:
As long as you find yourself not knowing what to test, you are not in a position to implement anything, yet. (Inconsistencies in specification tend to stand out in test design & implementation.)
Programming challenges à la leetcode.com typically build on simpler challenges - you met 2sum, which puts you in a favourable position to tackle 3sum.
The performance part of these challenges is about not doing things over, not discarding knowledge more often than about algebraic insight.
The generic solution to 2sum is to take values and find the complement (1sum?).
The extension to k-sum is to split k into smaller numbers.
Ways to have information about the order of a set or sequence (say, elements) of instances of a type with ordering reusable is to have them ordered (ordered = sorted(elements)
) or sorted (histogram = Counter(elements)
).
For 2sum, you could search for target/2
and work inside-out.
For 3sum, one value will be no greater, another no smaller than both others. The third one will not "lie outside" the former two.
$endgroup$
add a comment |
$begingroup$
Multi-part answer: [0) these preliminaries] 1) review of code presented 2) hints for improvement excluding personal, from programming over python and accepting a programming challenge to k-sum and leetcode 3Sum.
One general principle to follow is do as expected, in programming, it has been formulated in many guises, including Principle of Least Surprise. Every other route leaves you under pressure to justify, at risk of being misunderstood.
In coding, document, in the program source code:
What is "everything" there for?
Such rules often have been gathered into guide lines, for Python, follow the Style Guide for Python Code.
- Instead of a docstring, your module starts with imports - most of them unused.
If your code mentioned leetcode, the presentation of aclass Solution
with just one function definition wouldn't beg justification.- the definition of
threeSum()
shows (a cute addition oftarget
&default and) a curious mix of leetcode's templates for Python 2&3, lacking a proper docstring - comparing "the int"
target
to the list literal[0, 0, 0]
is dangerous given Python's zeal to allow operations: do you know by heart when that evaluates toTrue
?
(Do you expect most every reader of your code to?)
(Belatedly, it occurred to me that you may have intended to comparenums
to[0, 0, 0]
- bogus iftarget != 0
) - verbosity -
you can denote a tuple containing a single literal list like([v, v, v],)
- 2nd early out:
with only one key→value in the dict, there's no need to access it:
every key will equalnums[0]
You need to check for3*nums[0] != target
- naming (Not your fault:
Solution
andthreeSum
are substandard.nums
/_1-3 don't feel bad enough to change):
-target
,triplets
,sub_target
: right on!
- given Python's "duck typing", I'd stick withtriplets
(, never introducingtriplets_set
)
-lookup
is a horrible name →value_to_index
(or, in a suitably small context, justindex
)
(- there's one thing wrong withk
: it gets in the way when extending three_sum() to k_sum()…)
pythonic or not (or exploiting library functions, rather?):value_to_index = { value: index for index, value in enumerate(nums) }
(same forfor i, num_1 in enumerate(nums)
,for j, num_2 in enumerate(nums[i+1:], i+1)
)- commenting
- you did comment, and I find half of the comments helpful
- I don't get#overwrite from the high
(that may just be me)
-#don't reproduce itself
: probablyuse any element/index once, at most
rather thandon't repeat an element/index
- you did not comment the outer for loop, arguably the most profitable place:
how is what the execution constructs a solution?:
for each value,value_to_index
keeps just the last index: how does this not prevent any valid triple to show up in the solution? - checking
k
: nice!
(I'd go forin (None, i, j)
)
you don't provide a "tinker harness"
if __name__ == '__main__':
object_disoriented = Solution()
print(object_disoriented.threeSum([-1, 0, 1, 2, -1, -4]))
print(object_disoriented.threeSum([1, 1, 1], 3))
(Something else to follow is make (judicious) use of every help you can: if you use an IDE supporting Python, chances are it can help you with PEP8.)
(Running low on steam, this part will start more frugal than expected.)
Real Programming is the creation of a language that simplifies the solution of the problem at hand.
With Python providing most mechanisms needed in programming challenges, that leaves:
- Code readably.
- Create elements easy to use correctly.
I bickered you some about having a consistent problem description.
This is another place where Test First shines:
As long as you find yourself not knowing what to test, you are not in a position to implement anything, yet. (Inconsistencies in specification tend to stand out in test design & implementation.)
Programming challenges à la leetcode.com typically build on simpler challenges - you met 2sum, which puts you in a favourable position to tackle 3sum.
The performance part of these challenges is about not doing things over, not discarding knowledge more often than about algebraic insight.
The generic solution to 2sum is to take values and find the complement (1sum?).
The extension to k-sum is to split k into smaller numbers.
Ways to have information about the order of a set or sequence (say, elements) of instances of a type with ordering reusable is to have them ordered (ordered = sorted(elements)
) or sorted (histogram = Counter(elements)
).
For 2sum, you could search for target/2
and work inside-out.
For 3sum, one value will be no greater, another no smaller than both others. The third one will not "lie outside" the former two.
$endgroup$
add a comment |
$begingroup$
Multi-part answer: [0) these preliminaries] 1) review of code presented 2) hints for improvement excluding personal, from programming over python and accepting a programming challenge to k-sum and leetcode 3Sum.
One general principle to follow is do as expected, in programming, it has been formulated in many guises, including Principle of Least Surprise. Every other route leaves you under pressure to justify, at risk of being misunderstood.
In coding, document, in the program source code:
What is "everything" there for?
Such rules often have been gathered into guide lines, for Python, follow the Style Guide for Python Code.
- Instead of a docstring, your module starts with imports - most of them unused.
If your code mentioned leetcode, the presentation of aclass Solution
with just one function definition wouldn't beg justification.- the definition of
threeSum()
shows (a cute addition oftarget
&default and) a curious mix of leetcode's templates for Python 2&3, lacking a proper docstring - comparing "the int"
target
to the list literal[0, 0, 0]
is dangerous given Python's zeal to allow operations: do you know by heart when that evaluates toTrue
?
(Do you expect most every reader of your code to?)
(Belatedly, it occurred to me that you may have intended to comparenums
to[0, 0, 0]
- bogus iftarget != 0
) - verbosity -
you can denote a tuple containing a single literal list like([v, v, v],)
- 2nd early out:
with only one key→value in the dict, there's no need to access it:
every key will equalnums[0]
You need to check for3*nums[0] != target
- naming (Not your fault:
Solution
andthreeSum
are substandard.nums
/_1-3 don't feel bad enough to change):
-target
,triplets
,sub_target
: right on!
- given Python's "duck typing", I'd stick withtriplets
(, never introducingtriplets_set
)
-lookup
is a horrible name →value_to_index
(or, in a suitably small context, justindex
)
(- there's one thing wrong withk
: it gets in the way when extending three_sum() to k_sum()…)
pythonic or not (or exploiting library functions, rather?):value_to_index = { value: index for index, value in enumerate(nums) }
(same forfor i, num_1 in enumerate(nums)
,for j, num_2 in enumerate(nums[i+1:], i+1)
)- commenting
- you did comment, and I find half of the comments helpful
- I don't get#overwrite from the high
(that may just be me)
-#don't reproduce itself
: probablyuse any element/index once, at most
rather thandon't repeat an element/index
- you did not comment the outer for loop, arguably the most profitable place:
how is what the execution constructs a solution?:
for each value,value_to_index
keeps just the last index: how does this not prevent any valid triple to show up in the solution? - checking
k
: nice!
(I'd go forin (None, i, j)
)
you don't provide a "tinker harness"
if __name__ == '__main__':
object_disoriented = Solution()
print(object_disoriented.threeSum([-1, 0, 1, 2, -1, -4]))
print(object_disoriented.threeSum([1, 1, 1], 3))
(Something else to follow is make (judicious) use of every help you can: if you use an IDE supporting Python, chances are it can help you with PEP8.)
(Running low on steam, this part will start more frugal than expected.)
Real Programming is the creation of a language that simplifies the solution of the problem at hand.
With Python providing most mechanisms needed in programming challenges, that leaves:
- Code readably.
- Create elements easy to use correctly.
I bickered you some about having a consistent problem description.
This is another place where Test First shines:
As long as you find yourself not knowing what to test, you are not in a position to implement anything, yet. (Inconsistencies in specification tend to stand out in test design & implementation.)
Programming challenges à la leetcode.com typically build on simpler challenges - you met 2sum, which puts you in a favourable position to tackle 3sum.
The performance part of these challenges is about not doing things over, not discarding knowledge more often than about algebraic insight.
The generic solution to 2sum is to take values and find the complement (1sum?).
The extension to k-sum is to split k into smaller numbers.
Ways to have information about the order of a set or sequence (say, elements) of instances of a type with ordering reusable is to have them ordered (ordered = sorted(elements)
) or sorted (histogram = Counter(elements)
).
For 2sum, you could search for target/2
and work inside-out.
For 3sum, one value will be no greater, another no smaller than both others. The third one will not "lie outside" the former two.
$endgroup$
Multi-part answer: [0) these preliminaries] 1) review of code presented 2) hints for improvement excluding personal, from programming over python and accepting a programming challenge to k-sum and leetcode 3Sum.
One general principle to follow is do as expected, in programming, it has been formulated in many guises, including Principle of Least Surprise. Every other route leaves you under pressure to justify, at risk of being misunderstood.
In coding, document, in the program source code:
What is "everything" there for?
Such rules often have been gathered into guide lines, for Python, follow the Style Guide for Python Code.
- Instead of a docstring, your module starts with imports - most of them unused.
If your code mentioned leetcode, the presentation of aclass Solution
with just one function definition wouldn't beg justification.- the definition of
threeSum()
shows (a cute addition oftarget
&default and) a curious mix of leetcode's templates for Python 2&3, lacking a proper docstring - comparing "the int"
target
to the list literal[0, 0, 0]
is dangerous given Python's zeal to allow operations: do you know by heart when that evaluates toTrue
?
(Do you expect most every reader of your code to?)
(Belatedly, it occurred to me that you may have intended to comparenums
to[0, 0, 0]
- bogus iftarget != 0
) - verbosity -
you can denote a tuple containing a single literal list like([v, v, v],)
- 2nd early out:
with only one key→value in the dict, there's no need to access it:
every key will equalnums[0]
You need to check for3*nums[0] != target
- naming (Not your fault:
Solution
andthreeSum
are substandard.nums
/_1-3 don't feel bad enough to change):
-target
,triplets
,sub_target
: right on!
- given Python's "duck typing", I'd stick withtriplets
(, never introducingtriplets_set
)
-lookup
is a horrible name →value_to_index
(or, in a suitably small context, justindex
)
(- there's one thing wrong withk
: it gets in the way when extending three_sum() to k_sum()…)
pythonic or not (or exploiting library functions, rather?):value_to_index = { value: index for index, value in enumerate(nums) }
(same forfor i, num_1 in enumerate(nums)
,for j, num_2 in enumerate(nums[i+1:], i+1)
)- commenting
- you did comment, and I find half of the comments helpful
- I don't get#overwrite from the high
(that may just be me)
-#don't reproduce itself
: probablyuse any element/index once, at most
rather thandon't repeat an element/index
- you did not comment the outer for loop, arguably the most profitable place:
how is what the execution constructs a solution?:
for each value,value_to_index
keeps just the last index: how does this not prevent any valid triple to show up in the solution? - checking
k
: nice!
(I'd go forin (None, i, j)
)
you don't provide a "tinker harness"
if __name__ == '__main__':
object_disoriented = Solution()
print(object_disoriented.threeSum([-1, 0, 1, 2, -1, -4]))
print(object_disoriented.threeSum([1, 1, 1], 3))
(Something else to follow is make (judicious) use of every help you can: if you use an IDE supporting Python, chances are it can help you with PEP8.)
(Running low on steam, this part will start more frugal than expected.)
Real Programming is the creation of a language that simplifies the solution of the problem at hand.
With Python providing most mechanisms needed in programming challenges, that leaves:
- Code readably.
- Create elements easy to use correctly.
I bickered you some about having a consistent problem description.
This is another place where Test First shines:
As long as you find yourself not knowing what to test, you are not in a position to implement anything, yet. (Inconsistencies in specification tend to stand out in test design & implementation.)
Programming challenges à la leetcode.com typically build on simpler challenges - you met 2sum, which puts you in a favourable position to tackle 3sum.
The performance part of these challenges is about not doing things over, not discarding knowledge more often than about algebraic insight.
The generic solution to 2sum is to take values and find the complement (1sum?).
The extension to k-sum is to split k into smaller numbers.
Ways to have information about the order of a set or sequence (say, elements) of instances of a type with ordering reusable is to have them ordered (ordered = sorted(elements)
) or sorted (histogram = Counter(elements)
).
For 2sum, you could search for target/2
and work inside-out.
For 3sum, one value will be no greater, another no smaller than both others. The third one will not "lie outside" the former two.
answered yesterday
greybeardgreybeard
1,6291723
1,6291723
add a comment |
add a comment |
Alice is a new contributor. Be nice, and check out our Code of Conduct.
Alice is a new contributor. Be nice, and check out our Code of Conduct.
Alice is a new contributor. Be nice, and check out our Code of Conduct.
Alice is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216207%2fin-an-set-of-integers-find-three-elements-summing-to-zero-3-sum-leetcode-vari%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g2t7jy,EKm zmK,bb mW7Vq6j xefv L86lhn sh1MH,M P0Gh ayO22k19PiVWe hVlOm1Rq6Y8y
1
$begingroup$
Can you check the problem description? It is about pairs (a, b) which sum to 9, but the examples are about triples which sum to zero. – Btw, if you beat 28.86 of other submissions then you are slower 71.14 percent, not slower than 80 percent :)
$endgroup$
– Martin R
Mar 26 at 5:48
1
$begingroup$
As set is a common verb in English, try to avoid confusion: set is a Python built-in data type. (Grant me the favour and never call any amount of program source code "codes".) How could
target: int
== [0, 0, 0]
?$endgroup$
– greybeard
Mar 26 at 6:31
$begingroup$
amazing, better than leetcode's big data testing. @greybeard
$endgroup$
– Alice
Mar 26 at 10:25
$begingroup$
Ah, before I forget again: Congrats on asking for a review, for
hints to improve
instead of a turn-key solution, on (seemingly) trying to come up with a solution of your own instead of scraping the web/SE/leetcode. Way to learn!$endgroup$
– greybeard
yesterday