Efficient substring matchString Matching and ClusteringAutocomplete Trie OptimizationLongest increasing...
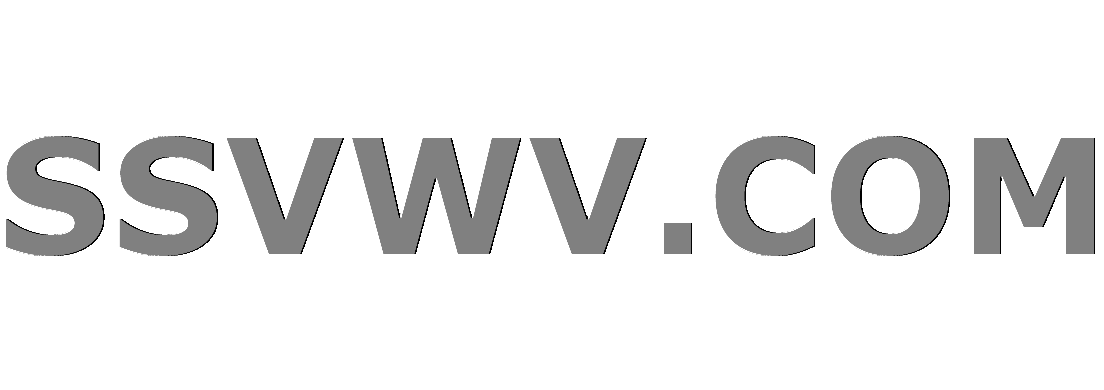
Multi tool use
How to acknowledge an embarrassing job interview, now that I work directly with the interviewer?
Can a person refuse a presidential pardon?
Slow moving projectiles from a hand-held weapon - how do they reach the target?
Can I write a book of my D&D game?
Could flying insects re-enter the Earth's atmosphere from space without burning up?
If I delete my router's history can my ISP still provide it to my parents?
It took me a lot of time to make this, pls like. (YouTube Comments #1)
Why avoid shared user accounts?
How to tag distinct options/entities without giving any an implicit priority or suggested order?
Cryptic with missing capitals
Can a dragon be stuck looking like a human?
Jumping Numbers
What makes the Forgotten Realms "forgotten"?
Do authors have to be politically correct in article-writing?
Strange Sign on Lab Door
Groups acting on trees
Disable the ">" operator in Rstudio linux terminal
Recrystallisation of dibenzylideneacetone
Does fast page mode apply to ROM?
Why did the villain in the first Men in Black movie care about Earth's Cockroaches?
Why does a metal block make a shrill sound but not a wooden block upon hammering?
What to do if authors don't respond to my serious concerns about their paper?
How to prevent users from executing commands through browser URL
Why did this image turn out darker?
Efficient substring match
String Matching and ClusteringAutocomplete Trie OptimizationLongest increasing subsequence and common substringLongest Palindromic SubstringFinding longest common prefixLongest common substring using dynamic programmingLargest substring which starts and ends with some substringPython program to check substring match locations with a lot of permutations of the substringThe Substring Game! challengeLongest substring in alphabetical order
$begingroup$
I have a list of unique strings (approx, 25,00,000) of different lengths and I am trying to find if there is any string which occurs as a substring of previous strings.
def index_containing_substring(the_list, substring):
for i, s in enumerate(the_list):
if substring in s:
return i
return -1
def string_match():
test_list=['foo bar abc xml','fdff gdnfgf gdkgf','foo bar','abc','xml','xyz']
max_len=4 # I am storing the maximum length of sentence
# the list starts with reverse order
# i.e sentence with highest length are at the top
safe_to_add=[]
for s in test_list:
if len(s)==max_len:
safe_to_add.append(s)
else:
idx=index_containing_substring(safe_to_add,s)
if idx==-1:
safe_to_add.append(s)
else:
# process the substring
print('match found {} for {}'.format(test_list[idx],s))
This method works fine but I think it is pretty slow. Is there a better way to solve this problem using a better data structure (trie or suffix tree)?
Output
match found foo bar abc xml for foo bar
match found foo bar abc xml for abc
match found foo bar abc xml for xml
python algorithm python-3.x strings
$endgroup$
add a comment |
$begingroup$
I have a list of unique strings (approx, 25,00,000) of different lengths and I am trying to find if there is any string which occurs as a substring of previous strings.
def index_containing_substring(the_list, substring):
for i, s in enumerate(the_list):
if substring in s:
return i
return -1
def string_match():
test_list=['foo bar abc xml','fdff gdnfgf gdkgf','foo bar','abc','xml','xyz']
max_len=4 # I am storing the maximum length of sentence
# the list starts with reverse order
# i.e sentence with highest length are at the top
safe_to_add=[]
for s in test_list:
if len(s)==max_len:
safe_to_add.append(s)
else:
idx=index_containing_substring(safe_to_add,s)
if idx==-1:
safe_to_add.append(s)
else:
# process the substring
print('match found {} for {}'.format(test_list[idx],s))
This method works fine but I think it is pretty slow. Is there a better way to solve this problem using a better data structure (trie or suffix tree)?
Output
match found foo bar abc xml for foo bar
match found foo bar abc xml for abc
match found foo bar abc xml for xml
python algorithm python-3.x strings
$endgroup$
$begingroup$
(25,00,000
looks off. It might help if you delimited the strings in the output likematch found in <foo bar abc xml> for foo bar
.)list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string wasab
: would a match be shown forabc
, too?
$endgroup$
– greybeard
2 hours ago
add a comment |
$begingroup$
I have a list of unique strings (approx, 25,00,000) of different lengths and I am trying to find if there is any string which occurs as a substring of previous strings.
def index_containing_substring(the_list, substring):
for i, s in enumerate(the_list):
if substring in s:
return i
return -1
def string_match():
test_list=['foo bar abc xml','fdff gdnfgf gdkgf','foo bar','abc','xml','xyz']
max_len=4 # I am storing the maximum length of sentence
# the list starts with reverse order
# i.e sentence with highest length are at the top
safe_to_add=[]
for s in test_list:
if len(s)==max_len:
safe_to_add.append(s)
else:
idx=index_containing_substring(safe_to_add,s)
if idx==-1:
safe_to_add.append(s)
else:
# process the substring
print('match found {} for {}'.format(test_list[idx],s))
This method works fine but I think it is pretty slow. Is there a better way to solve this problem using a better data structure (trie or suffix tree)?
Output
match found foo bar abc xml for foo bar
match found foo bar abc xml for abc
match found foo bar abc xml for xml
python algorithm python-3.x strings
$endgroup$
I have a list of unique strings (approx, 25,00,000) of different lengths and I am trying to find if there is any string which occurs as a substring of previous strings.
def index_containing_substring(the_list, substring):
for i, s in enumerate(the_list):
if substring in s:
return i
return -1
def string_match():
test_list=['foo bar abc xml','fdff gdnfgf gdkgf','foo bar','abc','xml','xyz']
max_len=4 # I am storing the maximum length of sentence
# the list starts with reverse order
# i.e sentence with highest length are at the top
safe_to_add=[]
for s in test_list:
if len(s)==max_len:
safe_to_add.append(s)
else:
idx=index_containing_substring(safe_to_add,s)
if idx==-1:
safe_to_add.append(s)
else:
# process the substring
print('match found {} for {}'.format(test_list[idx],s))
This method works fine but I think it is pretty slow. Is there a better way to solve this problem using a better data structure (trie or suffix tree)?
Output
match found foo bar abc xml for foo bar
match found foo bar abc xml for abc
match found foo bar abc xml for xml
python algorithm python-3.x strings
python algorithm python-3.x strings
edited 3 hours ago


Jamal♦
30.3k11119227
30.3k11119227
asked 3 hours ago
RohitRohit
54531020
54531020
$begingroup$
(25,00,000
looks off. It might help if you delimited the strings in the output likematch found in <foo bar abc xml> for foo bar
.)list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string wasab
: would a match be shown forabc
, too?
$endgroup$
– greybeard
2 hours ago
add a comment |
$begingroup$
(25,00,000
looks off. It might help if you delimited the strings in the output likematch found in <foo bar abc xml> for foo bar
.)list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string wasab
: would a match be shown forabc
, too?
$endgroup$
– greybeard
2 hours ago
$begingroup$
(
25,00,000
looks off. It might help if you delimited the strings in the output like match found in <foo bar abc xml> for foo bar
.) list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string was ab
: would a match be shown for abc
, too?$endgroup$
– greybeard
2 hours ago
$begingroup$
(
25,00,000
looks off. It might help if you delimited the strings in the output like match found in <foo bar abc xml> for foo bar
.) list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string was ab
: would a match be shown for abc
, too?$endgroup$
– greybeard
2 hours ago
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214587%2fefficient-substring-match%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214587%2fefficient-substring-match%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W 42gN,k,n
$begingroup$
(
25,00,000
looks off. It might help if you delimited the strings in the output likematch found in <foo bar abc xml> for foo bar
.)list starts [with] highest length
Is non-increasing length a guaranteed property of the input? What if the third string wasab
: would a match be shown forabc
, too?$endgroup$
– greybeard
2 hours ago