Tic Tac Toe Game in Java using MVC [closed]Detecting Tic-Tac-Toe win: Enumerate all possibilities or use...
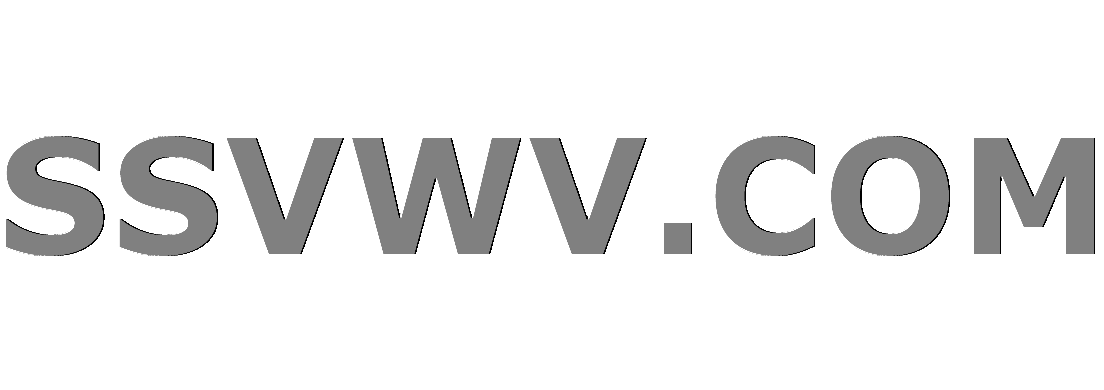
Multi tool use
A newer friend of my brother's gave him a load of baseball cards that are supposedly extremely valuable. Is this a scam?
The use of multiple foreign keys on same column in SQL Server
How to find program name(s) of an installed package?
Have astronauts in space suits ever taken selfies? If so, how?
How is the claim "I am in New York only if I am in America" the same as "If I am in New York, then I am in America?
Pattern match does not work in bash script
Did Shadowfax go to Valinor?
How is it possible to have an ability score that is less than 3?
Why does Kotter return in Welcome Back Kotter?
In Japanese, what’s the difference between “Tonari ni” (となりに) and “Tsugi” (つぎ)? When would you use one over the other?
Which models of the Boeing 737 are still in production?
Finding angle with pure Geometry.
"to be prejudice towards/against someone" vs "to be prejudiced against/towards someone"
Can divisibility rules for digits be generalized to sum of digits
Why was the small council so happy for Tyrion to become the Master of Coin?
Prove that NP is closed under karp reduction?
Why are 150k or 200k jobs considered good when there are 300k+ births a month?
Test if tikzmark exists on same page
How to add double frame in tcolorbox?
Mathematical cryptic clues
Does Unearthed Arcana render Favored Souls redundant?
Arthur Somervell: 1000 Exercises - Meaning of this notation
Approximately how much travel time was saved by the opening of the Suez Canal in 1869?
Why are electrically insulating heatsinks so rare? Is it just cost?
Tic Tac Toe Game in Java using MVC [closed]
Detecting Tic-Tac-Toe win: Enumerate all possibilities or use nested loops?Tic-tac-toe 'get winner' algorithmTic Tac Toe getWinner() method logic for a grid of any size nxnTic Tac Toe in JavaUltimate Tic Tac Toe A.K.A. Tic TacticsTic Tac T-OO: Design and ImplementationCallback-oriented Tic-Tac-ToeChecking for a win in Tic Tac ToeTic-Tac-Toe game simulator in JavaTic Tac Toe game - object oriented Java
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
$begingroup$
I'm working on a Tic Tac Toe game with java and need to implement it with the MVC pattern. Just wondering on what will be a good place to start? I have a working game currently only using java.
//Tic-Tac-Toe. Two player.
//Feel free to use as a starting point for 5x5 and separating into MVC.
import java.util.Scanner;
public class TicTacToe {
static final int X = 1;
static final int O = -1;
//printBoard method that prints out the current state of the
//Tic-Tac-Toe board. Does not return anything or change the board.
public static void printBoard( int [][] matrix ){
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[row].length; col++ ){
// Uses the "global" constants to print out appropriate letter.
if( matrix[row][col] == X )
System.out.print("X ");
else if(matrix[row][col] == O )
System.out.print("O ");
else
System.out.print(". ");
}
// Goes to new line after printing each row
System.out.println("");
}
}
//hasWon returns true if there was a win or a cat game.
public static boolean hasWon( int [][] matrix ){
//Variable holds the "result" of hasWon. True if a winner was found.
boolean retVal = false;
//Check for horizontal win
for( int row = 0; row < matrix.length; row++ ){
int sum = 0;
for( int col = 0; col < matrix[0].length; col++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that row was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for vertical win
for( int col = 0; col < matrix[0].length; col++ ){
int sum = 0;
for( int row = 0; row < matrix.length; row++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that column was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for diagonal win
if( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
if( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
//Check for cat game
boolean foundSpace = false;
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[0].length; col++ ){
if( matrix[row][col] == 0 )
foundSpace = true;
}
}
if( foundSpace == false ){
System.out.println("Ends in tie.");
retVal = true;
}
return retVal;
}
public static void main (String [] args) {
Scanner input = new Scanner(System.in);
//A 5x5 array stored as integers. X will be 1. O will be -1.
int [][] board = new int[5][5];
while( hasWon(board) == false){
//Get the X player input and make the change if not taken.
System.out.print("X,(from 0-4) enter a row: ");
int row = input.nextInt();
System.out.print("X,(from 0-4) enter a column: ");
int col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = X;
printBoard(board);
//Check to see if X's move won the game. If so, break out of game loop
if( hasWon(board) == true )
break;
//Get the O player input and make the change if not taken.
System.out.print("O, (from 0-4) enter a row: ");
row = input.nextInt();
System.out.print("O, (from 0-4) enter a column: ");
col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = O;
printBoard(board);
}
System.out.println("Game over.");
}
}
java mvc
$endgroup$
closed as off-topic by πάντα ῥεῖ, 200_success, Raystafarian, t3chb0t, Toby Speight Mar 29 at 8:19
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – 200_success, t3chb0t, Toby Speight
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
$begingroup$
I'm working on a Tic Tac Toe game with java and need to implement it with the MVC pattern. Just wondering on what will be a good place to start? I have a working game currently only using java.
//Tic-Tac-Toe. Two player.
//Feel free to use as a starting point for 5x5 and separating into MVC.
import java.util.Scanner;
public class TicTacToe {
static final int X = 1;
static final int O = -1;
//printBoard method that prints out the current state of the
//Tic-Tac-Toe board. Does not return anything or change the board.
public static void printBoard( int [][] matrix ){
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[row].length; col++ ){
// Uses the "global" constants to print out appropriate letter.
if( matrix[row][col] == X )
System.out.print("X ");
else if(matrix[row][col] == O )
System.out.print("O ");
else
System.out.print(". ");
}
// Goes to new line after printing each row
System.out.println("");
}
}
//hasWon returns true if there was a win or a cat game.
public static boolean hasWon( int [][] matrix ){
//Variable holds the "result" of hasWon. True if a winner was found.
boolean retVal = false;
//Check for horizontal win
for( int row = 0; row < matrix.length; row++ ){
int sum = 0;
for( int col = 0; col < matrix[0].length; col++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that row was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for vertical win
for( int col = 0; col < matrix[0].length; col++ ){
int sum = 0;
for( int row = 0; row < matrix.length; row++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that column was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for diagonal win
if( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
if( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
//Check for cat game
boolean foundSpace = false;
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[0].length; col++ ){
if( matrix[row][col] == 0 )
foundSpace = true;
}
}
if( foundSpace == false ){
System.out.println("Ends in tie.");
retVal = true;
}
return retVal;
}
public static void main (String [] args) {
Scanner input = new Scanner(System.in);
//A 5x5 array stored as integers. X will be 1. O will be -1.
int [][] board = new int[5][5];
while( hasWon(board) == false){
//Get the X player input and make the change if not taken.
System.out.print("X,(from 0-4) enter a row: ");
int row = input.nextInt();
System.out.print("X,(from 0-4) enter a column: ");
int col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = X;
printBoard(board);
//Check to see if X's move won the game. If so, break out of game loop
if( hasWon(board) == true )
break;
//Get the O player input and make the change if not taken.
System.out.print("O, (from 0-4) enter a row: ");
row = input.nextInt();
System.out.print("O, (from 0-4) enter a column: ");
col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = O;
printBoard(board);
}
System.out.println("Game over.");
}
}
java mvc
$endgroup$
closed as off-topic by πάντα ῥεῖ, 200_success, Raystafarian, t3chb0t, Toby Speight Mar 29 at 8:19
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – 200_success, t3chb0t, Toby Speight
If this question can be reworded to fit the rules in the help center, please edit the question.
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
3
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26
add a comment |
$begingroup$
I'm working on a Tic Tac Toe game with java and need to implement it with the MVC pattern. Just wondering on what will be a good place to start? I have a working game currently only using java.
//Tic-Tac-Toe. Two player.
//Feel free to use as a starting point for 5x5 and separating into MVC.
import java.util.Scanner;
public class TicTacToe {
static final int X = 1;
static final int O = -1;
//printBoard method that prints out the current state of the
//Tic-Tac-Toe board. Does not return anything or change the board.
public static void printBoard( int [][] matrix ){
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[row].length; col++ ){
// Uses the "global" constants to print out appropriate letter.
if( matrix[row][col] == X )
System.out.print("X ");
else if(matrix[row][col] == O )
System.out.print("O ");
else
System.out.print(". ");
}
// Goes to new line after printing each row
System.out.println("");
}
}
//hasWon returns true if there was a win or a cat game.
public static boolean hasWon( int [][] matrix ){
//Variable holds the "result" of hasWon. True if a winner was found.
boolean retVal = false;
//Check for horizontal win
for( int row = 0; row < matrix.length; row++ ){
int sum = 0;
for( int col = 0; col < matrix[0].length; col++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that row was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for vertical win
for( int col = 0; col < matrix[0].length; col++ ){
int sum = 0;
for( int row = 0; row < matrix.length; row++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that column was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for diagonal win
if( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
if( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
//Check for cat game
boolean foundSpace = false;
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[0].length; col++ ){
if( matrix[row][col] == 0 )
foundSpace = true;
}
}
if( foundSpace == false ){
System.out.println("Ends in tie.");
retVal = true;
}
return retVal;
}
public static void main (String [] args) {
Scanner input = new Scanner(System.in);
//A 5x5 array stored as integers. X will be 1. O will be -1.
int [][] board = new int[5][5];
while( hasWon(board) == false){
//Get the X player input and make the change if not taken.
System.out.print("X,(from 0-4) enter a row: ");
int row = input.nextInt();
System.out.print("X,(from 0-4) enter a column: ");
int col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = X;
printBoard(board);
//Check to see if X's move won the game. If so, break out of game loop
if( hasWon(board) == true )
break;
//Get the O player input and make the change if not taken.
System.out.print("O, (from 0-4) enter a row: ");
row = input.nextInt();
System.out.print("O, (from 0-4) enter a column: ");
col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = O;
printBoard(board);
}
System.out.println("Game over.");
}
}
java mvc
$endgroup$
I'm working on a Tic Tac Toe game with java and need to implement it with the MVC pattern. Just wondering on what will be a good place to start? I have a working game currently only using java.
//Tic-Tac-Toe. Two player.
//Feel free to use as a starting point for 5x5 and separating into MVC.
import java.util.Scanner;
public class TicTacToe {
static final int X = 1;
static final int O = -1;
//printBoard method that prints out the current state of the
//Tic-Tac-Toe board. Does not return anything or change the board.
public static void printBoard( int [][] matrix ){
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[row].length; col++ ){
// Uses the "global" constants to print out appropriate letter.
if( matrix[row][col] == X )
System.out.print("X ");
else if(matrix[row][col] == O )
System.out.print("O ");
else
System.out.print(". ");
}
// Goes to new line after printing each row
System.out.println("");
}
}
//hasWon returns true if there was a win or a cat game.
public static boolean hasWon( int [][] matrix ){
//Variable holds the "result" of hasWon. True if a winner was found.
boolean retVal = false;
//Check for horizontal win
for( int row = 0; row < matrix.length; row++ ){
int sum = 0;
for( int col = 0; col < matrix[0].length; col++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that row was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for vertical win
for( int col = 0; col < matrix[0].length; col++ ){
int sum = 0;
for( int row = 0; row < matrix.length; row++ ){
sum += matrix[row][col];
}
//Check to see if the sum of that column was 3 or -3, a win...
if( sum == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( sum == -5 ) {
System.out.println("O wins.");
retVal = true;
}
}
//Check for diagonal win
if( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][0] + matrix[1][1] + matrix[2][2] + matrix[3][3] + matrix[4][4]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
if( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == 5 ){
System.out.println("X wins.");
retVal = true;
} else if ( (matrix[0][4] + matrix[1][3] + matrix[2][2] + matrix[3][1] + matrix[4][0]) == -5 ) {
System.out.println("O wins.");
retVal = true;
}
//Check for cat game
boolean foundSpace = false;
for( int row = 0; row < matrix.length; row++ ){
for( int col = 0; col < matrix[0].length; col++ ){
if( matrix[row][col] == 0 )
foundSpace = true;
}
}
if( foundSpace == false ){
System.out.println("Ends in tie.");
retVal = true;
}
return retVal;
}
public static void main (String [] args) {
Scanner input = new Scanner(System.in);
//A 5x5 array stored as integers. X will be 1. O will be -1.
int [][] board = new int[5][5];
while( hasWon(board) == false){
//Get the X player input and make the change if not taken.
System.out.print("X,(from 0-4) enter a row: ");
int row = input.nextInt();
System.out.print("X,(from 0-4) enter a column: ");
int col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = X;
printBoard(board);
//Check to see if X's move won the game. If so, break out of game loop
if( hasWon(board) == true )
break;
//Get the O player input and make the change if not taken.
System.out.print("O, (from 0-4) enter a row: ");
row = input.nextInt();
System.out.print("O, (from 0-4) enter a column: ");
col = input.nextInt();
if( board[row][col] == 0 )
board[row][col] = O;
printBoard(board);
}
System.out.println("Game over.");
}
}
java mvc
java mvc
asked Mar 28 at 21:05
Robert WaguespackRobert Waguespack
1
1
closed as off-topic by πάντα ῥεῖ, 200_success, Raystafarian, t3chb0t, Toby Speight Mar 29 at 8:19
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – 200_success, t3chb0t, Toby Speight
If this question can be reworded to fit the rules in the help center, please edit the question.
closed as off-topic by πάντα ῥεῖ, 200_success, Raystafarian, t3chb0t, Toby Speight Mar 29 at 8:19
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – 200_success, t3chb0t, Toby Speight
If this question can be reworded to fit the rules in the help center, please edit the question.
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
3
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26
add a comment |
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
3
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
3
3
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26
add a comment |
0
active
oldest
votes
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
3Cp0iYS
$begingroup$
Welcome to Code Review! Code Review is intended to be used as a platform where people give feedback on your working and implemented code. It would be on-topic for this site to ask for a review on the code as is. Adding features or transforming your code into another pattern is not considered a valid question for code review.
$endgroup$
– Alex
Mar 28 at 21:17
3
$begingroup$
Since the code is not in any form of MVC, asking for it to be made into MVC is asking for code to be written (or a tutorial), which is off-topic for Code Review.
$endgroup$
– 200_success
Mar 29 at 5:26