A recursive solution to symmetirc treeCheck if a binary tree is a subtree of another treeAlgorithm that...
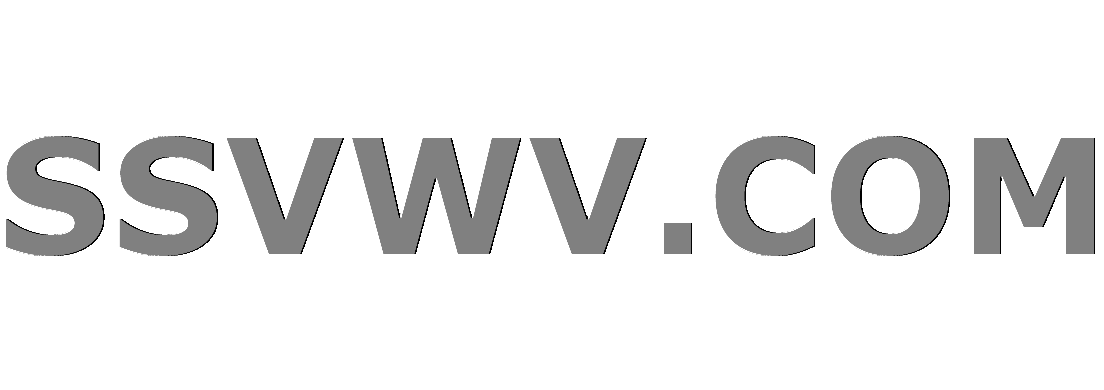
Multi tool use
What is the offset in a seaplane's hull?
Modification to Chariots for Heavy Cavalry Analogue for 4-armed race
What defenses are there against being summoned by the Gate spell?
Are tax years 2016 & 2017 back taxes deductible for tax year 2018?
Download, install and reboot computer at night if needed
least quadratic residue under GRH: an EXPLICIT bound
Email Account under attack (really) - anything I can do?
How to calculate implied correlation via observed market price (Margrabe option)
What do you call a Matrix-like slowdown and camera movement effect?
Are white and non-white police officers equally likely to kill black suspects?
How does one intimidate enemies without having the capacity for violence?
Why don't electron-positron collisions release infinite energy?
The use of multiple foreign keys on same column in SQL Server
Can Medicine checks be used, with decent rolls, to completely mitigate the risk of death from ongoing damage?
Extreme, but not acceptable situation and I can't start the work tomorrow morning
A function which translates a sentence to title-case
I see my dog run
Is it tax fraud for an individual to declare non-taxable revenue as taxable income? (US tax laws)
Is it possible to do 50 km distance without any previous training?
Is it possible to make sharp wind that can cut stuff from afar?
DOS, create pipe for stdin/stdout of command.com(or 4dos.com) in C or Batch?
How is the claim "I am in New York only if I am in America" the same as "If I am in New York, then I am in America?
Why is the design of haulage companies so “special”?
How to report a triplet of septets in NMR tabulation?
A recursive solution to symmetirc tree
Check if a binary tree is a subtree of another treeAlgorithm that checks if a tree is full and completeSearch iteratively in a ternary search treeGoogle Foobar Challenge: Ion Flux RelabelingCheck if two binary trees are equalJava: Given a list of edges, build a tree and return the rootGiven a binary tree, find the maximum path sumAt least 2 paths down the binary tree have the same sumGeneric binary search tree in C++Leetcode 102: Binary tree level-order traversal in Swift
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
$begingroup$
I tried to solve a symmetric tree problem
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).
For example, this binary tree
[1,2,2,3,4,4,3]
is symmetric:
1
/
2 2
/ /
3 4 4 3
But the following
[1,2,2,null,3,null,3]
is not:
1
/
2 2
3 3
Note:
Bonus points if you could solve it both recursively and iteratively.
My solution with recursion
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def isSymmetric(self, root):
if not root: return True #None is symmetic
return self.isMirror(root.left, root.right)
def isMirror(self, l, r):
if not l and not r: return True #base case 1
if not l or not r: return False #base case 2
if l.val != r.val: return False #base case 3
#recur case
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
I assumed it as a decent solution to this problem but get a low score
Runtime: 32 ms, faster than 24.42% of Python online submissions for Symmetric Tree.
Memory Usage: 12.2 MB, less than 5.08% of Python online submissions for Symmetric Tree.
How could improve the my solution?
python performance algorithm tree
$endgroup$
add a comment |
$begingroup$
I tried to solve a symmetric tree problem
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).
For example, this binary tree
[1,2,2,3,4,4,3]
is symmetric:
1
/
2 2
/ /
3 4 4 3
But the following
[1,2,2,null,3,null,3]
is not:
1
/
2 2
3 3
Note:
Bonus points if you could solve it both recursively and iteratively.
My solution with recursion
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def isSymmetric(self, root):
if not root: return True #None is symmetic
return self.isMirror(root.left, root.right)
def isMirror(self, l, r):
if not l and not r: return True #base case 1
if not l or not r: return False #base case 2
if l.val != r.val: return False #base case 3
#recur case
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
I assumed it as a decent solution to this problem but get a low score
Runtime: 32 ms, faster than 24.42% of Python online submissions for Symmetric Tree.
Memory Usage: 12.2 MB, less than 5.08% of Python online submissions for Symmetric Tree.
How could improve the my solution?
python performance algorithm tree
$endgroup$
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linkedTreeNode
objects?
$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37
add a comment |
$begingroup$
I tried to solve a symmetric tree problem
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).
For example, this binary tree
[1,2,2,3,4,4,3]
is symmetric:
1
/
2 2
/ /
3 4 4 3
But the following
[1,2,2,null,3,null,3]
is not:
1
/
2 2
3 3
Note:
Bonus points if you could solve it both recursively and iteratively.
My solution with recursion
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def isSymmetric(self, root):
if not root: return True #None is symmetic
return self.isMirror(root.left, root.right)
def isMirror(self, l, r):
if not l and not r: return True #base case 1
if not l or not r: return False #base case 2
if l.val != r.val: return False #base case 3
#recur case
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
I assumed it as a decent solution to this problem but get a low score
Runtime: 32 ms, faster than 24.42% of Python online submissions for Symmetric Tree.
Memory Usage: 12.2 MB, less than 5.08% of Python online submissions for Symmetric Tree.
How could improve the my solution?
python performance algorithm tree
$endgroup$
I tried to solve a symmetric tree problem
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).
For example, this binary tree
[1,2,2,3,4,4,3]
is symmetric:
1
/
2 2
/ /
3 4 4 3
But the following
[1,2,2,null,3,null,3]
is not:
1
/
2 2
3 3
Note:
Bonus points if you could solve it both recursively and iteratively.
My solution with recursion
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def isSymmetric(self, root):
if not root: return True #None is symmetic
return self.isMirror(root.left, root.right)
def isMirror(self, l, r):
if not l and not r: return True #base case 1
if not l or not r: return False #base case 2
if l.val != r.val: return False #base case 3
#recur case
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
I assumed it as a decent solution to this problem but get a low score
Runtime: 32 ms, faster than 24.42% of Python online submissions for Symmetric Tree.
Memory Usage: 12.2 MB, less than 5.08% of Python online submissions for Symmetric Tree.
How could improve the my solution?
python performance algorithm tree
python performance algorithm tree
edited Apr 1 at 12:42


200_success
131k17157422
131k17157422
asked Apr 1 at 10:36


AliceAlice
3206
3206
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linkedTreeNode
objects?
$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37
add a comment |
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linkedTreeNode
objects?
$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linked
TreeNode
objects?$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linked
TreeNode
objects?$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37
add a comment |
2 Answers
2
active
oldest
votes
$begingroup$
Possibly the most obvious part is here
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
there's no need to perform the second test if the first one returns False
:
if not self.isMirror(l.left, r.right): return False
if not self.isMirror(l.right, r.left): return False
return True
$endgroup$
add a comment |
$begingroup$
Appart from the optimization provided by @CiaPan, you could try using an inner function to reduce the need for attributes lookups and accelerate symbols resolution speed:
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
def isMirror(left, right):
if left is None and right is None:
return True
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
return isMirror(left.left, right.right) and isMirror(left.right, right.left)
return isMirror(root.left, root.right)
Alternatively, you could try the iterative approach which is usually implemented using a deque
to perform a breadth first search:
from collections import deque
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
rights = deque([root.right])
lefts = deque([root.left])
while lefts:
left = lefts.popleft()
right = right.popleft()
if left is None and right is None:
pass
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
lefts.append(left.left)
lefts.append(left.right)
rights.append(right.right)
rights.append(right.left)
return True
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216641%2fa-recursive-solution-to-symmetirc-tree%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Possibly the most obvious part is here
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
there's no need to perform the second test if the first one returns False
:
if not self.isMirror(l.left, r.right): return False
if not self.isMirror(l.right, r.left): return False
return True
$endgroup$
add a comment |
$begingroup$
Possibly the most obvious part is here
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
there's no need to perform the second test if the first one returns False
:
if not self.isMirror(l.left, r.right): return False
if not self.isMirror(l.right, r.left): return False
return True
$endgroup$
add a comment |
$begingroup$
Possibly the most obvious part is here
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
there's no need to perform the second test if the first one returns False
:
if not self.isMirror(l.left, r.right): return False
if not self.isMirror(l.right, r.left): return False
return True
$endgroup$
Possibly the most obvious part is here
left = self.isMirror(l.left, r.right)
right = self.isMirror(l.right, r.left)
return left and right
there's no need to perform the second test if the first one returns False
:
if not self.isMirror(l.left, r.right): return False
if not self.isMirror(l.right, r.left): return False
return True
answered Apr 1 at 11:01
CiaPanCiaPan
1,3971513
1,3971513
add a comment |
add a comment |
$begingroup$
Appart from the optimization provided by @CiaPan, you could try using an inner function to reduce the need for attributes lookups and accelerate symbols resolution speed:
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
def isMirror(left, right):
if left is None and right is None:
return True
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
return isMirror(left.left, right.right) and isMirror(left.right, right.left)
return isMirror(root.left, root.right)
Alternatively, you could try the iterative approach which is usually implemented using a deque
to perform a breadth first search:
from collections import deque
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
rights = deque([root.right])
lefts = deque([root.left])
while lefts:
left = lefts.popleft()
right = right.popleft()
if left is None and right is None:
pass
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
lefts.append(left.left)
lefts.append(left.right)
rights.append(right.right)
rights.append(right.left)
return True
$endgroup$
add a comment |
$begingroup$
Appart from the optimization provided by @CiaPan, you could try using an inner function to reduce the need for attributes lookups and accelerate symbols resolution speed:
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
def isMirror(left, right):
if left is None and right is None:
return True
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
return isMirror(left.left, right.right) and isMirror(left.right, right.left)
return isMirror(root.left, root.right)
Alternatively, you could try the iterative approach which is usually implemented using a deque
to perform a breadth first search:
from collections import deque
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
rights = deque([root.right])
lefts = deque([root.left])
while lefts:
left = lefts.popleft()
right = right.popleft()
if left is None and right is None:
pass
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
lefts.append(left.left)
lefts.append(left.right)
rights.append(right.right)
rights.append(right.left)
return True
$endgroup$
add a comment |
$begingroup$
Appart from the optimization provided by @CiaPan, you could try using an inner function to reduce the need for attributes lookups and accelerate symbols resolution speed:
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
def isMirror(left, right):
if left is None and right is None:
return True
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
return isMirror(left.left, right.right) and isMirror(left.right, right.left)
return isMirror(root.left, root.right)
Alternatively, you could try the iterative approach which is usually implemented using a deque
to perform a breadth first search:
from collections import deque
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
rights = deque([root.right])
lefts = deque([root.left])
while lefts:
left = lefts.popleft()
right = right.popleft()
if left is None and right is None:
pass
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
lefts.append(left.left)
lefts.append(left.right)
rights.append(right.right)
rights.append(right.left)
return True
$endgroup$
Appart from the optimization provided by @CiaPan, you could try using an inner function to reduce the need for attributes lookups and accelerate symbols resolution speed:
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
def isMirror(left, right):
if left is None and right is None:
return True
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
return isMirror(left.left, right.right) and isMirror(left.right, right.left)
return isMirror(root.left, root.right)
Alternatively, you could try the iterative approach which is usually implemented using a deque
to perform a breadth first search:
from collections import deque
class Solution(object):
def isSymmetric(self, root):
if root is None:
return True
rights = deque([root.right])
lefts = deque([root.left])
while lefts:
left = lefts.popleft()
right = right.popleft()
if left is None and right is None:
pass
elif left is None or right is None:
return False
elif left.val != right.val:
return False
else:
lefts.append(left.left)
lefts.append(left.right)
rights.append(right.right)
rights.append(right.left)
return True
answered Apr 1 at 17:01


Mathias EttingerMathias Ettinger
25.2k33386
25.2k33386
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216641%2fa-recursive-solution-to-symmetirc-tree%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p tFfrt4g1eP5QwLVGzyXeP25opsk4jBd7G4,QLM,LTHuEbcApzHej
$begingroup$
You present input data both as a list and as a tree. Which one is the actual input form? If it is a list of integers, possibly you could do a recursive analysis just on that list and save the time needed for constructing a tree of linked
TreeNode
objects?$endgroup$
– CiaPan
Apr 1 at 11:33
$begingroup$
You 'accepted' my answer, which should confirm my modification is correct and resolves your problem. If so, do you mind to share the scores your modified algorithm achieves?
$endgroup$
– CiaPan
Apr 1 at 12:46
$begingroup$
Where are you submitting these answers to get rankings like this?
$endgroup$
– Shawson
Apr 1 at 13:16
$begingroup$
@Shawson Possibly it's Geeks for geeks? Or may be the LeetCode?
$endgroup$
– CiaPan
Apr 1 at 17:37