How is it possible to add a double into an ArrayList of Integer? (Java)Java generics type erasure: when and...
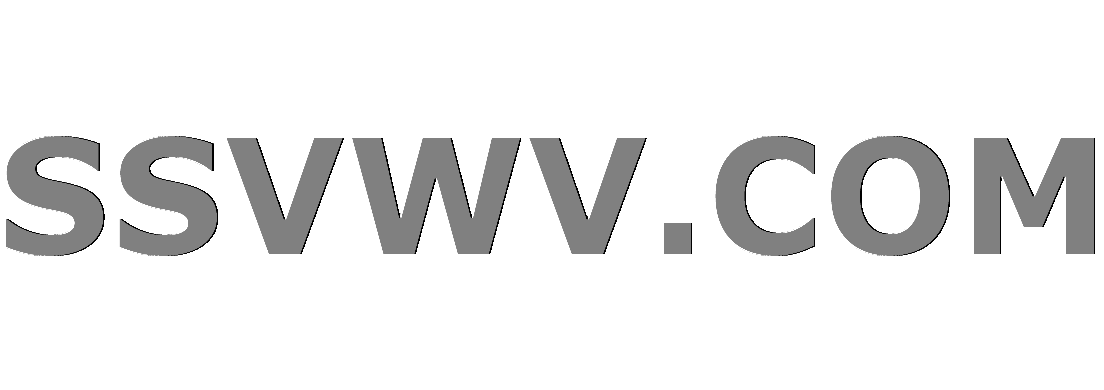
Multi tool use
Why use "finir par" instead of "finir de" before an infinitive?
What happens if you roll doubles 3 times then land on "Go to jail?"
Can someone clarify the logic behind the given equation?
Would a high gravity rocky planet be guaranteed to have an atmosphere?
How to write papers efficiently when English isn't my first language?
Applicability of Single Responsibility Principle
What do "Victor", "Tango", "Quebec", "Eco", "Sierra" flight ticket classes mean?
What can we do to stop prior company from asking us questions?
Rotate a column
What grammatical function is や performing here?
Hostile work environment after whistle-blowing on coworker and our boss. What do I do?
Lay out the Carpet
All SQL Server aliases linked to default instance instead of instance I assigned
How should I support this large drywall patch?
Anatomically Correct Strange Women In Ponds Distributing Swords
How do we know the LHC results are robust?
How do I go from 300 unfinished/half written blog posts, to published posts?
How do I extract a value from a time formatted value in excel?
Is the destination of a commercial flight important for the pilot?
What is the difference between "behavior" and "behaviour"?
I'm in charge of equipment buying but no one's ever happy with what I choose. How to fix this?
How can a function with a hole (removable discontinuity) equal a function with no hole?
Only print output after finding pattern
Sort a list by elements of another list
How is it possible to add a double into an ArrayList of Integer? (Java)
Java generics type erasure: when and what happens?type erasure in implementation of ArrayList in JavaHow do I efficiently iterate over each entry in a Java Map?How do I call one constructor from another in Java?How do I read / convert an InputStream into a String in Java?When to use LinkedList over ArrayList in Java?How do I generate random integers within a specific range in Java?How do I determine whether an array contains a particular value in Java?How do I declare and initialize an array in Java?How to split a string in JavaConverting 'ArrayList<String> to 'String[]' in JavaHow do I convert a String to an int in Java?
I try to understand how is it possible to have a Double
value into an ArrayList
of Integer
. The numList
is an ArrayList
of Integer
, and the value from it is a Double
.
This is the code:
package bounded.wildcards;
import java.util.ArrayList;
import java.util.List;
public class GenericsDemo {
public static void main(String[] args) {
// Invariance Workaround
List<Integer> numList = new ArrayList<>();
GenericsDemo.invarianceWorkaround(numList);
System.out.println(numList);
}
static <T extends Number> void invarianceWorkaround(List<T> list) {
T element = (T) new Double(23.3);
list.add(element);
}
}
This will compile and run without an error.
java generics arraylist
add a comment |
I try to understand how is it possible to have a Double
value into an ArrayList
of Integer
. The numList
is an ArrayList
of Integer
, and the value from it is a Double
.
This is the code:
package bounded.wildcards;
import java.util.ArrayList;
import java.util.List;
public class GenericsDemo {
public static void main(String[] args) {
// Invariance Workaround
List<Integer> numList = new ArrayList<>();
GenericsDemo.invarianceWorkaround(numList);
System.out.println(numList);
}
static <T extends Number> void invarianceWorkaround(List<T> list) {
T element = (T) new Double(23.3);
list.add(element);
}
}
This will compile and run without an error.
java generics arraylist
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago
add a comment |
I try to understand how is it possible to have a Double
value into an ArrayList
of Integer
. The numList
is an ArrayList
of Integer
, and the value from it is a Double
.
This is the code:
package bounded.wildcards;
import java.util.ArrayList;
import java.util.List;
public class GenericsDemo {
public static void main(String[] args) {
// Invariance Workaround
List<Integer> numList = new ArrayList<>();
GenericsDemo.invarianceWorkaround(numList);
System.out.println(numList);
}
static <T extends Number> void invarianceWorkaround(List<T> list) {
T element = (T) new Double(23.3);
list.add(element);
}
}
This will compile and run without an error.
java generics arraylist
I try to understand how is it possible to have a Double
value into an ArrayList
of Integer
. The numList
is an ArrayList
of Integer
, and the value from it is a Double
.
This is the code:
package bounded.wildcards;
import java.util.ArrayList;
import java.util.List;
public class GenericsDemo {
public static void main(String[] args) {
// Invariance Workaround
List<Integer> numList = new ArrayList<>();
GenericsDemo.invarianceWorkaround(numList);
System.out.println(numList);
}
static <T extends Number> void invarianceWorkaround(List<T> list) {
T element = (T) new Double(23.3);
list.add(element);
}
}
This will compile and run without an error.
java generics arraylist
java generics arraylist
edited 19 mins ago
gaby
asked 1 hour ago
gabygaby
48411
48411
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago
add a comment |
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago
add a comment |
1 Answer
1
active
oldest
votes
This is because of type erasure used with Java generics - the type checks are only performed at compile time for generic types, and the type info for generics is then erased, effectively turning List<Integer>
into List<Object>
.
My IDE warns you of an "Unchecked cast from Double to T". But the compiler couldn't be sure that your code is wrong, so it does not emit an error, just a warning.
Then at runtime, the type check is no longer present due to type erasure, so the code will run without error unless you perform some operation that fails due to incompatible runtime type. System.out.println()
is not such operation.
If you change the print code to
Integer num = numList.get(0);
System.out.println(num);
this will now involve runtime type check and will therefore fail:
java.lang.ClassCastException: java.lang.Double cannot be cast to java.lang.Integer
3
Note that aClassCastException
is emitted when one tries to do this:Integer i = numList.get(0)
.
– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already inNumber
.
– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning intoList<Number>
notList<Object>
because of upper bound isNumber
. if its List<Object> then you can store String but you can't
– Akash Shah
37 mins ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55396803%2fhow-is-it-possible-to-add-a-double-into-an-arraylist-of-integer-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is because of type erasure used with Java generics - the type checks are only performed at compile time for generic types, and the type info for generics is then erased, effectively turning List<Integer>
into List<Object>
.
My IDE warns you of an "Unchecked cast from Double to T". But the compiler couldn't be sure that your code is wrong, so it does not emit an error, just a warning.
Then at runtime, the type check is no longer present due to type erasure, so the code will run without error unless you perform some operation that fails due to incompatible runtime type. System.out.println()
is not such operation.
If you change the print code to
Integer num = numList.get(0);
System.out.println(num);
this will now involve runtime type check and will therefore fail:
java.lang.ClassCastException: java.lang.Double cannot be cast to java.lang.Integer
3
Note that aClassCastException
is emitted when one tries to do this:Integer i = numList.get(0)
.
– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already inNumber
.
– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning intoList<Number>
notList<Object>
because of upper bound isNumber
. if its List<Object> then you can store String but you can't
– Akash Shah
37 mins ago
add a comment |
This is because of type erasure used with Java generics - the type checks are only performed at compile time for generic types, and the type info for generics is then erased, effectively turning List<Integer>
into List<Object>
.
My IDE warns you of an "Unchecked cast from Double to T". But the compiler couldn't be sure that your code is wrong, so it does not emit an error, just a warning.
Then at runtime, the type check is no longer present due to type erasure, so the code will run without error unless you perform some operation that fails due to incompatible runtime type. System.out.println()
is not such operation.
If you change the print code to
Integer num = numList.get(0);
System.out.println(num);
this will now involve runtime type check and will therefore fail:
java.lang.ClassCastException: java.lang.Double cannot be cast to java.lang.Integer
3
Note that aClassCastException
is emitted when one tries to do this:Integer i = numList.get(0)
.
– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already inNumber
.
– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning intoList<Number>
notList<Object>
because of upper bound isNumber
. if its List<Object> then you can store String but you can't
– Akash Shah
37 mins ago
add a comment |
This is because of type erasure used with Java generics - the type checks are only performed at compile time for generic types, and the type info for generics is then erased, effectively turning List<Integer>
into List<Object>
.
My IDE warns you of an "Unchecked cast from Double to T". But the compiler couldn't be sure that your code is wrong, so it does not emit an error, just a warning.
Then at runtime, the type check is no longer present due to type erasure, so the code will run without error unless you perform some operation that fails due to incompatible runtime type. System.out.println()
is not such operation.
If you change the print code to
Integer num = numList.get(0);
System.out.println(num);
this will now involve runtime type check and will therefore fail:
java.lang.ClassCastException: java.lang.Double cannot be cast to java.lang.Integer
This is because of type erasure used with Java generics - the type checks are only performed at compile time for generic types, and the type info for generics is then erased, effectively turning List<Integer>
into List<Object>
.
My IDE warns you of an "Unchecked cast from Double to T". But the compiler couldn't be sure that your code is wrong, so it does not emit an error, just a warning.
Then at runtime, the type check is no longer present due to type erasure, so the code will run without error unless you perform some operation that fails due to incompatible runtime type. System.out.println()
is not such operation.
If you change the print code to
Integer num = numList.get(0);
System.out.println(num);
this will now involve runtime type check and will therefore fail:
java.lang.ClassCastException: java.lang.Double cannot be cast to java.lang.Integer
edited 1 hour ago
answered 1 hour ago


Jiri TousekJiri Tousek
10.5k52240
10.5k52240
3
Note that aClassCastException
is emitted when one tries to do this:Integer i = numList.get(0)
.
– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already inNumber
.
– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning intoList<Number>
notList<Object>
because of upper bound isNumber
. if its List<Object> then you can store String but you can't
– Akash Shah
37 mins ago
add a comment |
3
Note that aClassCastException
is emitted when one tries to do this:Integer i = numList.get(0)
.
– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already inNumber
.
– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning intoList<Number>
notList<Object>
because of upper bound isNumber
. if its List<Object> then you can store String but you can't
– Akash Shah
37 mins ago
3
3
Note that a
ClassCastException
is emitted when one tries to do this: Integer i = numList.get(0)
.– MC Emperor
1 hour ago
Note that a
ClassCastException
is emitted when one tries to do this: Integer i = numList.get(0)
.– MC Emperor
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already in
Number
.– Jiri Tousek
1 hour ago
@MCEmperor Thanks, added. I couldn't find a simple example to force type incompatibility - integer seems to basically have no meaningful methods that wouldn't either be static or already in
Number
.– Jiri Tousek
1 hour ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
@JiriTousek You may be interested in Java is Unsound. Basically all java compilers will add cast checks in places to avoid issues because they know that the type system is broken.
– Giacomo Alzetta
45 mins ago
effectively turning into
List<Number>
not List<Object>
because of upper bound is Number
. if its List<Object> then you can store String but you can't– Akash Shah
37 mins ago
effectively turning into
List<Number>
not List<Object>
because of upper bound is Number
. if its List<Object> then you can store String but you can't– Akash Shah
37 mins ago
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55396803%2fhow-is-it-possible-to-add-a-double-into-an-arraylist-of-integer-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Z UzcGaxC,mxmJPUWppuuTH7vphr5,3VSggFjUd,DJJk2 Qu yKDWtw
Possible duplicate of type erasure in implementation of ArrayList in Java
– vaxquis
18 mins ago
also, stackoverflow.com/questions/339699/… and many others... I think that someone can find an exact duplicate, but they are are extremely closely related.
– vaxquis
17 mins ago