Include Serilog sink requiring dependency before dependency injection in .NET CoreDependency injection and...
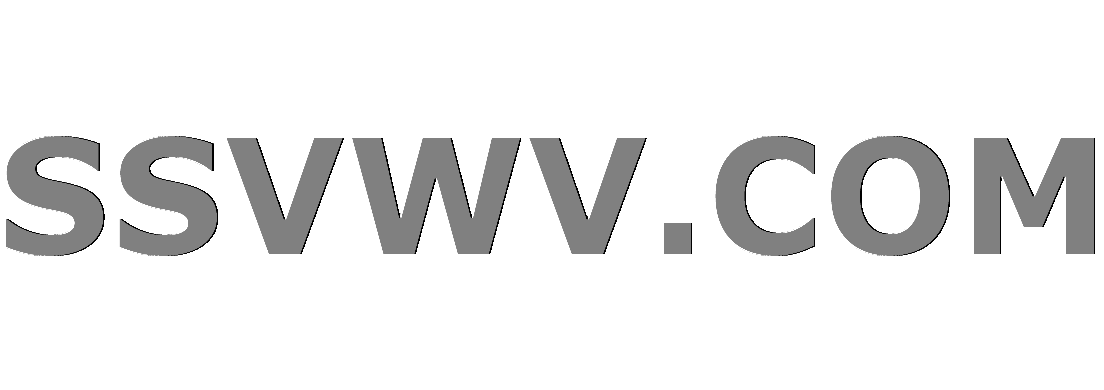
Multi tool use
How to write papers efficiently when English isn't my first language?
Was a professor correct to chastise me for writing "Prof. X" rather than "Professor X"?
Grabbing quick drinks
Failed to fetch jessie backports repository
What is the best translation for "slot" in the context of multiplayer video games?
Roman Numeral Treatment of Suspensions
How to create a 32-bit integer from eight (8) 4-bit integers?
Unreliable Magic - Is it worth it?
Is a stroke of luck acceptable after a series of unfavorable events?
How does the UK government determine the size of a mandate?
Opposite of a diet
In place solution to remove duplicates from a sorted list
Only print output after finding pattern
Is HostGator storing my password in plaintext?
'Given that' in a matrix
Describing a person. What needs to be mentioned?
Why are there no referendums in the US?
Return of the Riley Riddles in Reverse
Are there languages with no euphemisms?
Where does the Z80 processor start executing from?
How can I get through very long and very dry, but also very useful technical documents when learning a new tool?
Number of words that can be made using all the letters of the word W, if Os as well as Is are separated is?
Sort a list by elements of another list
Go Pregnant or Go Home
Include Serilog sink requiring dependency before dependency injection in .NET Core
Dependency injection and inversion of controlMVC N-Tier ArchitectureFactory dependency injectorRepository Pattern with Dapper + UnityUnit test Web API controller that uses a static helper class which uses app config settingRefactoring duplicate custom service configuration in .NET coreUnitOfWork for sending mailActivator with simple dependency injectionRetrieving OS Platform .NET CoreAuto register Func<T> for .net core dependecy injection
$begingroup$
Here is a rundown of the situation
- This is a .Net Core (2.1) application with a console and web front ends.
For simplicity this question focuses only on the console end although
the web end has the same issues.
Serilog is the logger of choice. We use 2 sinks (i.e. output sources): Console and MongoDB
- Security is a concern - the MongoDB connection string contains the password so it needs to be fetched from a secure source. Here we are using an custom created library to retrieve any sensitive information.
- My understanding is that Serilog configurations are not mutable (for example, add/configure another sink) after initialization, but can be modified after the fact to some extent with "enrichers". This is an aspect I am unfamiliar with.
- This solution is using standard .NET dependency injection
What's a better way of setting up Serilog, where a sink has a dependency, in a solution using dependency injection? Examples, patterns, templates are welcome. Most examples I've come across of Serilog with .NET Core DI don't address this situation.
public class Program
{
static int Main(string[] args)
{
var secrets = new ThirdPartySecretProvider("MyApp.Console");
var secretWrapper = new ThirdPartySecretProviderWrapper(secrets);
var loggingConnectionString = GetLoggingConnectionString(secretsWrapper);
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug()
.MinimumLevel.Override("Microsoft", LogEventLevel.Error)
.Enrich.FromLogContext()
.WriteTo.Console()
.WriteTo.MongoDB(loggingConnectionString, "applicationLogs")
.CreateLogger();
SelfLog.Enable(System.Console.Error);
var serviceCollection = new ServiceCollection();
ConfigureServices(serviceCollection, secretServerWrapper);
var serviceProvider = serviceCollection.BuildServiceProvider();
try
{
var logger = serviceProvider.GetRequiredService<ILoggerFactory>().CreateLogger<Application>();
var config = serviceProvider.GetRequiredService<IConfiguration>();
Application app = new Application(logger, config);
app.Run(args);
return 0;
}
catch (Exception ex)
{
Log.Fatal(ex, "Host terminated unexpectedly");
return 1;
}
finally
{
Log.CloseAndFlush();
}
}
private static void ConfigureServices(IServiceCollection services, ISecretsWrapper secrets)
{
IConfiguration config = new ConfigurationBuilder()
.AddJsonFile("appsettings.json", true, true)
.Build();
var mongoUsernameRead = config["MongoDB:ReadUsername"];
var mongoClientRead = new DataAccess.Mongo.MongoClient(mongoUsernameRead, secrets.MongoReadPassword);
services.AddSingleton<DataAccess.Mongo.IMongoClient>(mongoClientRead);
var hadoopRepository = new HadoopRepository(secretServer.HadoopApiKey);
services.AddSingleton<IHadoopRepository>(hadoopRepository);
services
.AddLogging(configure => configure.AddSerilog(dispose: true))
.AddSingleton(config)
.AddSingleton<ISecretsWrapper>(secrets)
.AddSingleton<IFileHelper, FileHelper>()
.AddSingleton<IRepositoryMongo, RepositoryMongo>()
.AddSingleton<IServiceMongo, ServiceMongo>()
.AddSingleton<IBaseHttpClient, BaseHttpClient>()
// ... additional dependencies omitted
}
private static string GetLoggingConnectionString(ISecretsWrapper secrets)
{
var mongoUsernameReadWrite = "app_myAppReadWrite";
var mongoClientLog = new DataAccess.Mongo.MongoClient(mongoUsernameReadWrite, secrets.MongoReadWritePassword);
return mongoClientLog.MongoConnectionString;
}
}
Related examples:
- Writeup by nblumhardt, a major Serilog contriutor https://nblumhardt.com/2017/08/use-serilog/
- Clean example with some a sink other than console https://jacksowter.net/serilog-config/
Stack Overflow example of creating multiple loggers at startup
Vanilla DI with .NET Core from MSDN
One potential change is to create a simple logger (using Serilogger or other) initially to capture failures before the application proper gets started, then create the desired logger to use for the rest of the application. What are the considerations of this approach?
c# logging dependency-injection .net-core
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
Here is a rundown of the situation
- This is a .Net Core (2.1) application with a console and web front ends.
For simplicity this question focuses only on the console end although
the web end has the same issues.
Serilog is the logger of choice. We use 2 sinks (i.e. output sources): Console and MongoDB
- Security is a concern - the MongoDB connection string contains the password so it needs to be fetched from a secure source. Here we are using an custom created library to retrieve any sensitive information.
- My understanding is that Serilog configurations are not mutable (for example, add/configure another sink) after initialization, but can be modified after the fact to some extent with "enrichers". This is an aspect I am unfamiliar with.
- This solution is using standard .NET dependency injection
What's a better way of setting up Serilog, where a sink has a dependency, in a solution using dependency injection? Examples, patterns, templates are welcome. Most examples I've come across of Serilog with .NET Core DI don't address this situation.
public class Program
{
static int Main(string[] args)
{
var secrets = new ThirdPartySecretProvider("MyApp.Console");
var secretWrapper = new ThirdPartySecretProviderWrapper(secrets);
var loggingConnectionString = GetLoggingConnectionString(secretsWrapper);
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug()
.MinimumLevel.Override("Microsoft", LogEventLevel.Error)
.Enrich.FromLogContext()
.WriteTo.Console()
.WriteTo.MongoDB(loggingConnectionString, "applicationLogs")
.CreateLogger();
SelfLog.Enable(System.Console.Error);
var serviceCollection = new ServiceCollection();
ConfigureServices(serviceCollection, secretServerWrapper);
var serviceProvider = serviceCollection.BuildServiceProvider();
try
{
var logger = serviceProvider.GetRequiredService<ILoggerFactory>().CreateLogger<Application>();
var config = serviceProvider.GetRequiredService<IConfiguration>();
Application app = new Application(logger, config);
app.Run(args);
return 0;
}
catch (Exception ex)
{
Log.Fatal(ex, "Host terminated unexpectedly");
return 1;
}
finally
{
Log.CloseAndFlush();
}
}
private static void ConfigureServices(IServiceCollection services, ISecretsWrapper secrets)
{
IConfiguration config = new ConfigurationBuilder()
.AddJsonFile("appsettings.json", true, true)
.Build();
var mongoUsernameRead = config["MongoDB:ReadUsername"];
var mongoClientRead = new DataAccess.Mongo.MongoClient(mongoUsernameRead, secrets.MongoReadPassword);
services.AddSingleton<DataAccess.Mongo.IMongoClient>(mongoClientRead);
var hadoopRepository = new HadoopRepository(secretServer.HadoopApiKey);
services.AddSingleton<IHadoopRepository>(hadoopRepository);
services
.AddLogging(configure => configure.AddSerilog(dispose: true))
.AddSingleton(config)
.AddSingleton<ISecretsWrapper>(secrets)
.AddSingleton<IFileHelper, FileHelper>()
.AddSingleton<IRepositoryMongo, RepositoryMongo>()
.AddSingleton<IServiceMongo, ServiceMongo>()
.AddSingleton<IBaseHttpClient, BaseHttpClient>()
// ... additional dependencies omitted
}
private static string GetLoggingConnectionString(ISecretsWrapper secrets)
{
var mongoUsernameReadWrite = "app_myAppReadWrite";
var mongoClientLog = new DataAccess.Mongo.MongoClient(mongoUsernameReadWrite, secrets.MongoReadWritePassword);
return mongoClientLog.MongoConnectionString;
}
}
Related examples:
- Writeup by nblumhardt, a major Serilog contriutor https://nblumhardt.com/2017/08/use-serilog/
- Clean example with some a sink other than console https://jacksowter.net/serilog-config/
Stack Overflow example of creating multiple loggers at startup
Vanilla DI with .NET Core from MSDN
One potential change is to create a simple logger (using Serilogger or other) initially to capture failures before the application proper gets started, then create the desired logger to use for the rest of the application. What are the considerations of this approach?
c# logging dependency-injection .net-core
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
Here is a rundown of the situation
- This is a .Net Core (2.1) application with a console and web front ends.
For simplicity this question focuses only on the console end although
the web end has the same issues.
Serilog is the logger of choice. We use 2 sinks (i.e. output sources): Console and MongoDB
- Security is a concern - the MongoDB connection string contains the password so it needs to be fetched from a secure source. Here we are using an custom created library to retrieve any sensitive information.
- My understanding is that Serilog configurations are not mutable (for example, add/configure another sink) after initialization, but can be modified after the fact to some extent with "enrichers". This is an aspect I am unfamiliar with.
- This solution is using standard .NET dependency injection
What's a better way of setting up Serilog, where a sink has a dependency, in a solution using dependency injection? Examples, patterns, templates are welcome. Most examples I've come across of Serilog with .NET Core DI don't address this situation.
public class Program
{
static int Main(string[] args)
{
var secrets = new ThirdPartySecretProvider("MyApp.Console");
var secretWrapper = new ThirdPartySecretProviderWrapper(secrets);
var loggingConnectionString = GetLoggingConnectionString(secretsWrapper);
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug()
.MinimumLevel.Override("Microsoft", LogEventLevel.Error)
.Enrich.FromLogContext()
.WriteTo.Console()
.WriteTo.MongoDB(loggingConnectionString, "applicationLogs")
.CreateLogger();
SelfLog.Enable(System.Console.Error);
var serviceCollection = new ServiceCollection();
ConfigureServices(serviceCollection, secretServerWrapper);
var serviceProvider = serviceCollection.BuildServiceProvider();
try
{
var logger = serviceProvider.GetRequiredService<ILoggerFactory>().CreateLogger<Application>();
var config = serviceProvider.GetRequiredService<IConfiguration>();
Application app = new Application(logger, config);
app.Run(args);
return 0;
}
catch (Exception ex)
{
Log.Fatal(ex, "Host terminated unexpectedly");
return 1;
}
finally
{
Log.CloseAndFlush();
}
}
private static void ConfigureServices(IServiceCollection services, ISecretsWrapper secrets)
{
IConfiguration config = new ConfigurationBuilder()
.AddJsonFile("appsettings.json", true, true)
.Build();
var mongoUsernameRead = config["MongoDB:ReadUsername"];
var mongoClientRead = new DataAccess.Mongo.MongoClient(mongoUsernameRead, secrets.MongoReadPassword);
services.AddSingleton<DataAccess.Mongo.IMongoClient>(mongoClientRead);
var hadoopRepository = new HadoopRepository(secretServer.HadoopApiKey);
services.AddSingleton<IHadoopRepository>(hadoopRepository);
services
.AddLogging(configure => configure.AddSerilog(dispose: true))
.AddSingleton(config)
.AddSingleton<ISecretsWrapper>(secrets)
.AddSingleton<IFileHelper, FileHelper>()
.AddSingleton<IRepositoryMongo, RepositoryMongo>()
.AddSingleton<IServiceMongo, ServiceMongo>()
.AddSingleton<IBaseHttpClient, BaseHttpClient>()
// ... additional dependencies omitted
}
private static string GetLoggingConnectionString(ISecretsWrapper secrets)
{
var mongoUsernameReadWrite = "app_myAppReadWrite";
var mongoClientLog = new DataAccess.Mongo.MongoClient(mongoUsernameReadWrite, secrets.MongoReadWritePassword);
return mongoClientLog.MongoConnectionString;
}
}
Related examples:
- Writeup by nblumhardt, a major Serilog contriutor https://nblumhardt.com/2017/08/use-serilog/
- Clean example with some a sink other than console https://jacksowter.net/serilog-config/
Stack Overflow example of creating multiple loggers at startup
Vanilla DI with .NET Core from MSDN
One potential change is to create a simple logger (using Serilogger or other) initially to capture failures before the application proper gets started, then create the desired logger to use for the rest of the application. What are the considerations of this approach?
c# logging dependency-injection .net-core
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
Here is a rundown of the situation
- This is a .Net Core (2.1) application with a console and web front ends.
For simplicity this question focuses only on the console end although
the web end has the same issues.
Serilog is the logger of choice. We use 2 sinks (i.e. output sources): Console and MongoDB
- Security is a concern - the MongoDB connection string contains the password so it needs to be fetched from a secure source. Here we are using an custom created library to retrieve any sensitive information.
- My understanding is that Serilog configurations are not mutable (for example, add/configure another sink) after initialization, but can be modified after the fact to some extent with "enrichers". This is an aspect I am unfamiliar with.
- This solution is using standard .NET dependency injection
What's a better way of setting up Serilog, where a sink has a dependency, in a solution using dependency injection? Examples, patterns, templates are welcome. Most examples I've come across of Serilog with .NET Core DI don't address this situation.
public class Program
{
static int Main(string[] args)
{
var secrets = new ThirdPartySecretProvider("MyApp.Console");
var secretWrapper = new ThirdPartySecretProviderWrapper(secrets);
var loggingConnectionString = GetLoggingConnectionString(secretsWrapper);
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug()
.MinimumLevel.Override("Microsoft", LogEventLevel.Error)
.Enrich.FromLogContext()
.WriteTo.Console()
.WriteTo.MongoDB(loggingConnectionString, "applicationLogs")
.CreateLogger();
SelfLog.Enable(System.Console.Error);
var serviceCollection = new ServiceCollection();
ConfigureServices(serviceCollection, secretServerWrapper);
var serviceProvider = serviceCollection.BuildServiceProvider();
try
{
var logger = serviceProvider.GetRequiredService<ILoggerFactory>().CreateLogger<Application>();
var config = serviceProvider.GetRequiredService<IConfiguration>();
Application app = new Application(logger, config);
app.Run(args);
return 0;
}
catch (Exception ex)
{
Log.Fatal(ex, "Host terminated unexpectedly");
return 1;
}
finally
{
Log.CloseAndFlush();
}
}
private static void ConfigureServices(IServiceCollection services, ISecretsWrapper secrets)
{
IConfiguration config = new ConfigurationBuilder()
.AddJsonFile("appsettings.json", true, true)
.Build();
var mongoUsernameRead = config["MongoDB:ReadUsername"];
var mongoClientRead = new DataAccess.Mongo.MongoClient(mongoUsernameRead, secrets.MongoReadPassword);
services.AddSingleton<DataAccess.Mongo.IMongoClient>(mongoClientRead);
var hadoopRepository = new HadoopRepository(secretServer.HadoopApiKey);
services.AddSingleton<IHadoopRepository>(hadoopRepository);
services
.AddLogging(configure => configure.AddSerilog(dispose: true))
.AddSingleton(config)
.AddSingleton<ISecretsWrapper>(secrets)
.AddSingleton<IFileHelper, FileHelper>()
.AddSingleton<IRepositoryMongo, RepositoryMongo>()
.AddSingleton<IServiceMongo, ServiceMongo>()
.AddSingleton<IBaseHttpClient, BaseHttpClient>()
// ... additional dependencies omitted
}
private static string GetLoggingConnectionString(ISecretsWrapper secrets)
{
var mongoUsernameReadWrite = "app_myAppReadWrite";
var mongoClientLog = new DataAccess.Mongo.MongoClient(mongoUsernameReadWrite, secrets.MongoReadWritePassword);
return mongoClientLog.MongoConnectionString;
}
}
Related examples:
- Writeup by nblumhardt, a major Serilog contriutor https://nblumhardt.com/2017/08/use-serilog/
- Clean example with some a sink other than console https://jacksowter.net/serilog-config/
Stack Overflow example of creating multiple loggers at startup
Vanilla DI with .NET Core from MSDN
One potential change is to create a simple logger (using Serilogger or other) initially to capture failures before the application proper gets started, then create the desired logger to use for the rest of the application. What are the considerations of this approach?
c# logging dependency-injection .net-core
c# logging dependency-injection .net-core
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 2 mins ago


ElsaElsa
12
12
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Elsa is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Elsa is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216417%2finclude-serilog-sink-requiring-dependency-before-dependency-injection-in-net-co%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Elsa is a new contributor. Be nice, and check out our Code of Conduct.
Elsa is a new contributor. Be nice, and check out our Code of Conduct.
Elsa is a new contributor. Be nice, and check out our Code of Conduct.
Elsa is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216417%2finclude-serilog-sink-requiring-dependency-before-dependency-injection-in-net-co%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rR7Dh5o9x4mt5 EYvUcI9Kme9kUriTeMm NIy6gWPSP 4nc,lhsZPD,V 0iji4