Non-blocking priority URL queue in PythonGeneric Task Blocking QueueUsing a Java queuePriority blocking queue...
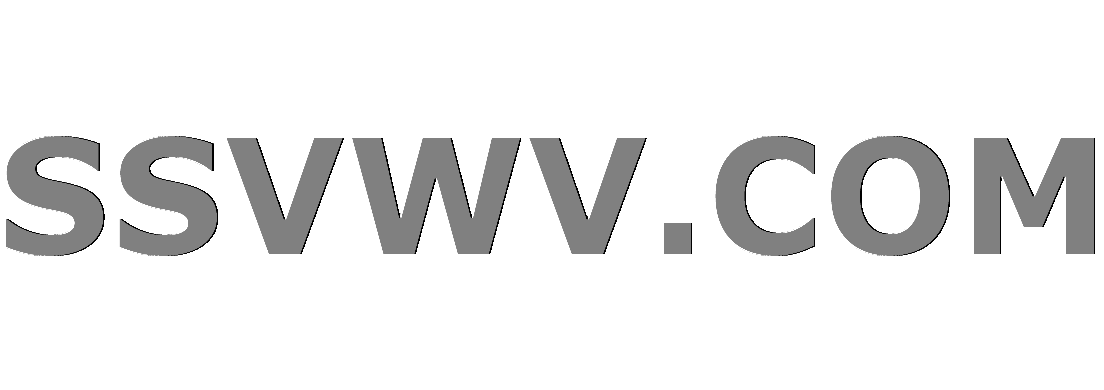
Multi tool use
In the late 1940’s to early 1950’s what technology was available that could melt a LOT of ice?
Can someone explain what is being said here in color publishing in the American Mathematical Monthly?
How much stiffer are 23c tires over 28c?
Why doesn't this Google Translate ad use the word "Translation" instead of "Translate"?
Am I not good enough for you?
Is it possible to have an Abelian group under two different binary operations but the binary operations are not distributive?
Single word request: Harming the benefactor
What to do when during a meeting client people start to fight (even physically) with each others?
How did the power source of Mar-Vell's aircraft end up with her?
Word for a person who has no opinion about whether god exists
Is there a window switcher for GNOME that shows the actual window?
Rejected in 4th interview round citing insufficient years of experience
If the Captain's screens are out, does he switch seats with the co-pilot?
Moving plot label
Fourth person (in Slavey language)
Are the terms "stab" and "staccato" synonyms?
Reverse string, can I make it faster?
Is it true that real estate prices mainly go up?
How do I express some one as a black person?
What Happens when Passenger Refuses to Fly Boeing 737 Max?
What are some noteworthy "mic-drop" moments in math?
Regex for certain words causes Spaces
What wound would be of little consequence to a biped but terrible for a quadruped?
Who deserves to be first and second author? PhD student who collected data, research associate who wrote the paper or supervisor?
Non-blocking priority URL queue in Python
Generic Task Blocking QueueUsing a Java queuePriority blocking queue implementationMultithreaded scoreboard for different levelsUpdatable priority queueBlocking and non-blocking queuePython priority queue class wrapperConcurrent FIFO in C++11Setup ordering in priority blocking queueA Priority Queue implemented using a Linked List
$begingroup$
I am trying to build a priority queue that never blocks on put()
, and discards the lowest priority item if it is full and user attempts to put()
a higher priority item. I will be using it as a URL queue for a web crawler, where the queue scores represent relevance to a search query (so that more relevant sites are fetched first).
Here is the code:
class URLQueue(Queue):
"""Non-blocking priority queue that tosses lowest priority (highest score) item when full, if attempt to add higher priority item ..."""
def _init(self, maxsize): # this overrides queue methods, see cpython/Lib/queue.py
self.queue = sortedcontainers.SortedList()
def _get_nowait(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def _get(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def __drop(self):
# drop the last (least important) item from the queue
# no consumer will get a chance to process this item, so
# we must decrement the unfinished count ourselves
dropped = self.queue.pop()
self.task_done()
return dropped # in case we need to add it back (if item we're attempting to put has higher score
def _put(self, item):
self.queue.add(item)
def put_nowait(self, new_item):
"""
compare the new_item we are attempting to add to last item in
queue (worst score), if item we're attempting to add has
better score, put it on queue, otherwise put previous max
item back on queue and drop new item
"""
try:
super().put_nowait(new_item)
except Full:
lowest_scored_item = self.__drop()
if new_item[0] > lowest_scored_item[0]:
super().put_nowait(lowest_scored_item)
else:
super().put_nowait(new_item)
def put(self, item):
# this queue never blocks, so we can call put_nowait() directly
super().put(item, block=False)
I am interested in any feedback/advice, but specifically I'm wondering:
Is there a better way to do this than using
sortedcontainers
to represent the internal queue? Is there a way that I could implement something using only builtins and standard library functions?Will the way I've done this cause any issues with threading? I will be putting/getting from the queue from multiple threads, and don't know if the way this is done will break something ...
Are there alternative data structures I could consider for maintaining a URL queue that prioritizes links based on some type of "relevance score"?
Thanks!
NOTE: I would like to give credit for the original idea of how to do this to this answer.
python multithreading sorting queue priority-queue
$endgroup$
add a comment |
$begingroup$
I am trying to build a priority queue that never blocks on put()
, and discards the lowest priority item if it is full and user attempts to put()
a higher priority item. I will be using it as a URL queue for a web crawler, where the queue scores represent relevance to a search query (so that more relevant sites are fetched first).
Here is the code:
class URLQueue(Queue):
"""Non-blocking priority queue that tosses lowest priority (highest score) item when full, if attempt to add higher priority item ..."""
def _init(self, maxsize): # this overrides queue methods, see cpython/Lib/queue.py
self.queue = sortedcontainers.SortedList()
def _get_nowait(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def _get(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def __drop(self):
# drop the last (least important) item from the queue
# no consumer will get a chance to process this item, so
# we must decrement the unfinished count ourselves
dropped = self.queue.pop()
self.task_done()
return dropped # in case we need to add it back (if item we're attempting to put has higher score
def _put(self, item):
self.queue.add(item)
def put_nowait(self, new_item):
"""
compare the new_item we are attempting to add to last item in
queue (worst score), if item we're attempting to add has
better score, put it on queue, otherwise put previous max
item back on queue and drop new item
"""
try:
super().put_nowait(new_item)
except Full:
lowest_scored_item = self.__drop()
if new_item[0] > lowest_scored_item[0]:
super().put_nowait(lowest_scored_item)
else:
super().put_nowait(new_item)
def put(self, item):
# this queue never blocks, so we can call put_nowait() directly
super().put(item, block=False)
I am interested in any feedback/advice, but specifically I'm wondering:
Is there a better way to do this than using
sortedcontainers
to represent the internal queue? Is there a way that I could implement something using only builtins and standard library functions?Will the way I've done this cause any issues with threading? I will be putting/getting from the queue from multiple threads, and don't know if the way this is done will break something ...
Are there alternative data structures I could consider for maintaining a URL queue that prioritizes links based on some type of "relevance score"?
Thanks!
NOTE: I would like to give credit for the original idea of how to do this to this answer.
python multithreading sorting queue priority-queue
$endgroup$
add a comment |
$begingroup$
I am trying to build a priority queue that never blocks on put()
, and discards the lowest priority item if it is full and user attempts to put()
a higher priority item. I will be using it as a URL queue for a web crawler, where the queue scores represent relevance to a search query (so that more relevant sites are fetched first).
Here is the code:
class URLQueue(Queue):
"""Non-blocking priority queue that tosses lowest priority (highest score) item when full, if attempt to add higher priority item ..."""
def _init(self, maxsize): # this overrides queue methods, see cpython/Lib/queue.py
self.queue = sortedcontainers.SortedList()
def _get_nowait(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def _get(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def __drop(self):
# drop the last (least important) item from the queue
# no consumer will get a chance to process this item, so
# we must decrement the unfinished count ourselves
dropped = self.queue.pop()
self.task_done()
return dropped # in case we need to add it back (if item we're attempting to put has higher score
def _put(self, item):
self.queue.add(item)
def put_nowait(self, new_item):
"""
compare the new_item we are attempting to add to last item in
queue (worst score), if item we're attempting to add has
better score, put it on queue, otherwise put previous max
item back on queue and drop new item
"""
try:
super().put_nowait(new_item)
except Full:
lowest_scored_item = self.__drop()
if new_item[0] > lowest_scored_item[0]:
super().put_nowait(lowest_scored_item)
else:
super().put_nowait(new_item)
def put(self, item):
# this queue never blocks, so we can call put_nowait() directly
super().put(item, block=False)
I am interested in any feedback/advice, but specifically I'm wondering:
Is there a better way to do this than using
sortedcontainers
to represent the internal queue? Is there a way that I could implement something using only builtins and standard library functions?Will the way I've done this cause any issues with threading? I will be putting/getting from the queue from multiple threads, and don't know if the way this is done will break something ...
Are there alternative data structures I could consider for maintaining a URL queue that prioritizes links based on some type of "relevance score"?
Thanks!
NOTE: I would like to give credit for the original idea of how to do this to this answer.
python multithreading sorting queue priority-queue
$endgroup$
I am trying to build a priority queue that never blocks on put()
, and discards the lowest priority item if it is full and user attempts to put()
a higher priority item. I will be using it as a URL queue for a web crawler, where the queue scores represent relevance to a search query (so that more relevant sites are fetched first).
Here is the code:
class URLQueue(Queue):
"""Non-blocking priority queue that tosses lowest priority (highest score) item when full, if attempt to add higher priority item ..."""
def _init(self, maxsize): # this overrides queue methods, see cpython/Lib/queue.py
self.queue = sortedcontainers.SortedList()
def _get_nowait(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def _get(self):
# pop the first (most important) item off the queue
return self.queue.pop(0)
def __drop(self):
# drop the last (least important) item from the queue
# no consumer will get a chance to process this item, so
# we must decrement the unfinished count ourselves
dropped = self.queue.pop()
self.task_done()
return dropped # in case we need to add it back (if item we're attempting to put has higher score
def _put(self, item):
self.queue.add(item)
def put_nowait(self, new_item):
"""
compare the new_item we are attempting to add to last item in
queue (worst score), if item we're attempting to add has
better score, put it on queue, otherwise put previous max
item back on queue and drop new item
"""
try:
super().put_nowait(new_item)
except Full:
lowest_scored_item = self.__drop()
if new_item[0] > lowest_scored_item[0]:
super().put_nowait(lowest_scored_item)
else:
super().put_nowait(new_item)
def put(self, item):
# this queue never blocks, so we can call put_nowait() directly
super().put(item, block=False)
I am interested in any feedback/advice, but specifically I'm wondering:
Is there a better way to do this than using
sortedcontainers
to represent the internal queue? Is there a way that I could implement something using only builtins and standard library functions?Will the way I've done this cause any issues with threading? I will be putting/getting from the queue from multiple threads, and don't know if the way this is done will break something ...
Are there alternative data structures I could consider for maintaining a URL queue that prioritizes links based on some type of "relevance score"?
Thanks!
NOTE: I would like to give credit for the original idea of how to do this to this answer.
python multithreading sorting queue priority-queue
python multithreading sorting queue priority-queue
asked 18 mins ago


J. TaylorJ. Taylor
1116
1116
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215308%2fnon-blocking-priority-url-queue-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215308%2fnon-blocking-priority-url-queue-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3yAipWzG7W8fQ,daKK7LrM28yEDGvM6uoqlS,Yit0,YdsH1dzaVI 6v7wZCi5MCykTW uUBnxP6zKtyRMoVlO,KdfGGzL6MrL