two arrays and random permutationsJava implementation of the longest monotone increasing...
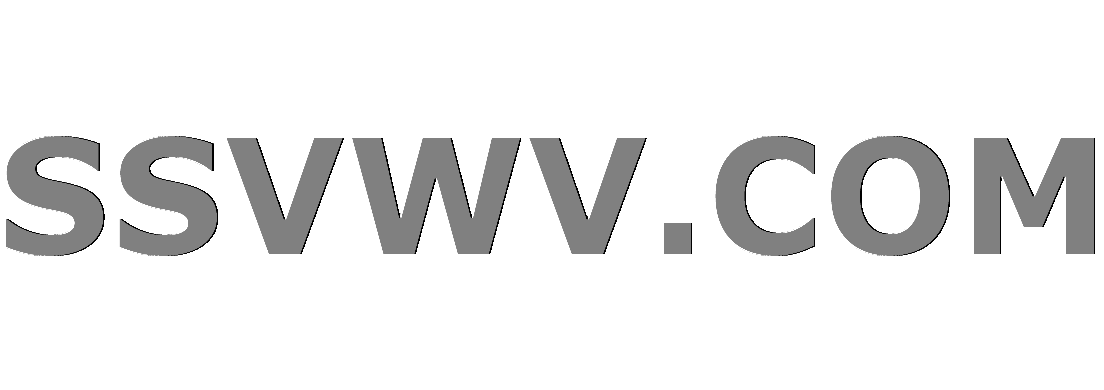
Multi tool use
Is there an elementary proof that there are infinitely many primes that are *not* completely split in an abelian extension?
Why don't MCU characters ever seem to have language issues?
Why doesn't this Google Translate ad use the word "Translation" instead of "Translate"?
What are actual Tesla M60 models used by AWS?
Virginia employer terminated employee and wants signing bonus returned
How to clip a background including nodes according to an arbitrary shape?
2000s TV show: people stuck in primitive other world, bit of magic and bit of dinosaurs
Why is there a voltage between the mains ground and my radiator?
How did Alan Turing break the enigma code using the hint given by the lady in the bar?
How can I ensure my trip to the UK will not have to be cancelled because of Brexit?
Peter's Strange Word
Tricky AM-GM inequality
Why would one plane in this picture not have gear down yet?
Why does Deadpool say "You're welcome, Canada," after shooting Ryan Reynolds in the end credits?
Who deserves to be first and second author? PhD student who collected data, research associate who wrote the paper or supervisor?
Force user to remove USB token
Low budget alien movie about the Earth being cooked
Built-In Shelves/Bookcases - IKEA vs Built
Who is eating data? Xargs?
Do f-stop and exposure time perfectly cancel?
Could you please stop shuffling the deck and play already?
What are some noteworthy "mic-drop" moments in math?
How much stiffer are 23c tires over 28c?
Making a sword in the stone, in a medieval world without magic
two arrays and random permutations
Java implementation of the longest monotone increasing subsequenceCalculating and displaying number statisticsRandom number wrapper classIntro to Java - determine valid unique numbersStreaming CollatzChecking if a number fits in a primitive typeFind prime positioned prime numberGuess number gameTesting a Random number generatorGCD of several integers
$begingroup$
First time poster here, very new to programming. Its for a Homework for a Java class i'm taking, I can not figure it out for the life of me. I understand there are probably a lot of errors in the code, but my primary focus is to get the program working without incorperating alot of high level knowledge The problem im trying to solve is as follows
" Write a program that displays a random permutation of the numbers 1 to 10.
The resulting permutation is stored in an array of integers. To generate a random permutation, you need
Hint: Use two arrays:
- One filled with the numbers in sequence 1-10 (this is your Array1)
- Then create a second array (Array2) initialized with 0 that you fill replace with the 0-10 numbers
of Array1 using the following algorithm:
Generate a random number in the range 0-9 that you use to represent the index (position) in Array1.
Copy the number found at that position in Array2.
You must keep track of the values already entered in Array2 since duplicates are not permitted
(this means that every time you try to enter a new number in Array2,
you must traverse Array2 to check if the number is already present. If it is present you try again
using a while loop. It it is not present, you append it to the values already entered.
Repeat 10 times.".
Here is the code I have thus far, please excuse typos that may be in comments.
//method for generating range of int numbers. Max is included in range.
public static int getRandomIntRange(int min, int max) {
int x = (int)(Math.random() * ((max + 1 - min) + 1)) + min;
if (x > (max + 1))
x = max;
return x;
}
public static void main(String[] args) {
//loop for storing 1-10 int number withing A1[].
int[] A1 = new int[10];
int count = 1;
for (int k = 0; k<A1.length; k++) {
A1[k] =count;
count++;;
}
// loop to test A1 is in sequence.
//for (int k = 0; k < 10 ; k++) {
//System.out.println(A1[k]);
//}
int [] A2 = new int[10];
int ranNum;
//loop to give A1 value to A2 with no repeats.
for(int k=0;k<A2.length; k++){
ranNum=getRandomIntRange(0,9);
if ((A2[0]!=A1[ranNum] )&&(A2[1] !=A1[ranNum])&&
(A2[2]!=A1[ranNum])&&(A2[3]!=A1[ranNum])&&
(A2[4]!=A1[ranNum])&&(A2[5]!=A1[ranNum])&&
(A2[6]!=A1[ranNum])&&(A2[7]!=A1[ranNum])&&(A2[8]!=A1[ranNum])&&(A2[9]!=A1[ranNum])) {
A2[k]=A1[ranNum];
}
}
for(int k =0; k<A2.length; k++) {
System.out.println(A2[k]);
}
}
}
java
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
First time poster here, very new to programming. Its for a Homework for a Java class i'm taking, I can not figure it out for the life of me. I understand there are probably a lot of errors in the code, but my primary focus is to get the program working without incorperating alot of high level knowledge The problem im trying to solve is as follows
" Write a program that displays a random permutation of the numbers 1 to 10.
The resulting permutation is stored in an array of integers. To generate a random permutation, you need
Hint: Use two arrays:
- One filled with the numbers in sequence 1-10 (this is your Array1)
- Then create a second array (Array2) initialized with 0 that you fill replace with the 0-10 numbers
of Array1 using the following algorithm:
Generate a random number in the range 0-9 that you use to represent the index (position) in Array1.
Copy the number found at that position in Array2.
You must keep track of the values already entered in Array2 since duplicates are not permitted
(this means that every time you try to enter a new number in Array2,
you must traverse Array2 to check if the number is already present. If it is present you try again
using a while loop. It it is not present, you append it to the values already entered.
Repeat 10 times.".
Here is the code I have thus far, please excuse typos that may be in comments.
//method for generating range of int numbers. Max is included in range.
public static int getRandomIntRange(int min, int max) {
int x = (int)(Math.random() * ((max + 1 - min) + 1)) + min;
if (x > (max + 1))
x = max;
return x;
}
public static void main(String[] args) {
//loop for storing 1-10 int number withing A1[].
int[] A1 = new int[10];
int count = 1;
for (int k = 0; k<A1.length; k++) {
A1[k] =count;
count++;;
}
// loop to test A1 is in sequence.
//for (int k = 0; k < 10 ; k++) {
//System.out.println(A1[k]);
//}
int [] A2 = new int[10];
int ranNum;
//loop to give A1 value to A2 with no repeats.
for(int k=0;k<A2.length; k++){
ranNum=getRandomIntRange(0,9);
if ((A2[0]!=A1[ranNum] )&&(A2[1] !=A1[ranNum])&&
(A2[2]!=A1[ranNum])&&(A2[3]!=A1[ranNum])&&
(A2[4]!=A1[ranNum])&&(A2[5]!=A1[ranNum])&&
(A2[6]!=A1[ranNum])&&(A2[7]!=A1[ranNum])&&(A2[8]!=A1[ranNum])&&(A2[9]!=A1[ranNum])) {
A2[k]=A1[ranNum];
}
}
for(int k =0; k<A2.length; k++) {
System.out.println(A2[k]);
}
}
}
java
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
First time poster here, very new to programming. Its for a Homework for a Java class i'm taking, I can not figure it out for the life of me. I understand there are probably a lot of errors in the code, but my primary focus is to get the program working without incorperating alot of high level knowledge The problem im trying to solve is as follows
" Write a program that displays a random permutation of the numbers 1 to 10.
The resulting permutation is stored in an array of integers. To generate a random permutation, you need
Hint: Use two arrays:
- One filled with the numbers in sequence 1-10 (this is your Array1)
- Then create a second array (Array2) initialized with 0 that you fill replace with the 0-10 numbers
of Array1 using the following algorithm:
Generate a random number in the range 0-9 that you use to represent the index (position) in Array1.
Copy the number found at that position in Array2.
You must keep track of the values already entered in Array2 since duplicates are not permitted
(this means that every time you try to enter a new number in Array2,
you must traverse Array2 to check if the number is already present. If it is present you try again
using a while loop. It it is not present, you append it to the values already entered.
Repeat 10 times.".
Here is the code I have thus far, please excuse typos that may be in comments.
//method for generating range of int numbers. Max is included in range.
public static int getRandomIntRange(int min, int max) {
int x = (int)(Math.random() * ((max + 1 - min) + 1)) + min;
if (x > (max + 1))
x = max;
return x;
}
public static void main(String[] args) {
//loop for storing 1-10 int number withing A1[].
int[] A1 = new int[10];
int count = 1;
for (int k = 0; k<A1.length; k++) {
A1[k] =count;
count++;;
}
// loop to test A1 is in sequence.
//for (int k = 0; k < 10 ; k++) {
//System.out.println(A1[k]);
//}
int [] A2 = new int[10];
int ranNum;
//loop to give A1 value to A2 with no repeats.
for(int k=0;k<A2.length; k++){
ranNum=getRandomIntRange(0,9);
if ((A2[0]!=A1[ranNum] )&&(A2[1] !=A1[ranNum])&&
(A2[2]!=A1[ranNum])&&(A2[3]!=A1[ranNum])&&
(A2[4]!=A1[ranNum])&&(A2[5]!=A1[ranNum])&&
(A2[6]!=A1[ranNum])&&(A2[7]!=A1[ranNum])&&(A2[8]!=A1[ranNum])&&(A2[9]!=A1[ranNum])) {
A2[k]=A1[ranNum];
}
}
for(int k =0; k<A2.length; k++) {
System.out.println(A2[k]);
}
}
}
java
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
First time poster here, very new to programming. Its for a Homework for a Java class i'm taking, I can not figure it out for the life of me. I understand there are probably a lot of errors in the code, but my primary focus is to get the program working without incorperating alot of high level knowledge The problem im trying to solve is as follows
" Write a program that displays a random permutation of the numbers 1 to 10.
The resulting permutation is stored in an array of integers. To generate a random permutation, you need
Hint: Use two arrays:
- One filled with the numbers in sequence 1-10 (this is your Array1)
- Then create a second array (Array2) initialized with 0 that you fill replace with the 0-10 numbers
of Array1 using the following algorithm:
Generate a random number in the range 0-9 that you use to represent the index (position) in Array1.
Copy the number found at that position in Array2.
You must keep track of the values already entered in Array2 since duplicates are not permitted
(this means that every time you try to enter a new number in Array2,
you must traverse Array2 to check if the number is already present. If it is present you try again
using a while loop. It it is not present, you append it to the values already entered.
Repeat 10 times.".
Here is the code I have thus far, please excuse typos that may be in comments.
//method for generating range of int numbers. Max is included in range.
public static int getRandomIntRange(int min, int max) {
int x = (int)(Math.random() * ((max + 1 - min) + 1)) + min;
if (x > (max + 1))
x = max;
return x;
}
public static void main(String[] args) {
//loop for storing 1-10 int number withing A1[].
int[] A1 = new int[10];
int count = 1;
for (int k = 0; k<A1.length; k++) {
A1[k] =count;
count++;;
}
// loop to test A1 is in sequence.
//for (int k = 0; k < 10 ; k++) {
//System.out.println(A1[k]);
//}
int [] A2 = new int[10];
int ranNum;
//loop to give A1 value to A2 with no repeats.
for(int k=0;k<A2.length; k++){
ranNum=getRandomIntRange(0,9);
if ((A2[0]!=A1[ranNum] )&&(A2[1] !=A1[ranNum])&&
(A2[2]!=A1[ranNum])&&(A2[3]!=A1[ranNum])&&
(A2[4]!=A1[ranNum])&&(A2[5]!=A1[ranNum])&&
(A2[6]!=A1[ranNum])&&(A2[7]!=A1[ranNum])&&(A2[8]!=A1[ranNum])&&(A2[9]!=A1[ranNum])) {
A2[k]=A1[ranNum];
}
}
for(int k =0; k<A2.length; k++) {
System.out.println(A2[k]);
}
}
}
java
java
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 5 mins ago
PRDPRD
1
1
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
PRD is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
PRD is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215287%2ftwo-arrays-and-random-permutations%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
PRD is a new contributor. Be nice, and check out our Code of Conduct.
PRD is a new contributor. Be nice, and check out our Code of Conduct.
PRD is a new contributor. Be nice, and check out our Code of Conduct.
PRD is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215287%2ftwo-arrays-and-random-permutations%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
j,GrrCBCQB0ZcbbEupfY7 NN2